STL (Stereolithography) format is a simple, openly documented format for describing the surface of an object as a triangular mesh. Every triangle is represented by the unit normal and vertices (ordered by the right-hand rule) using a three-dimensional Cartesian coordinate system.
There are two ways to export geometry to STL format:
- Using the STLExport module
- Using OdDb3dSolid
Exporting to STL using the STLExport Module
Exporting using the module is flexible, for example, you can export several solids or the whole model space to one .stl file. The export module file has the following name:
TD_STLExport_xx.yy_zz.tx
where:
- xx — Major Drawings SDK version number.
- yy — Minor Drawings SDK version number.
- zz — Microsoft® Visual C++® compiler version number. For example, for Microsoft Visual Studio® 2015 version, the number is 14.
To export using the STLExport module:
- Load the STLExport module.
- Create an OdDbBlockReference.
- Fill the created OdDbBlockReference with the solids and use one of the following functions:
- OdResult exportSTL(OdDbBaseDatabase *pDb, const OdGiDrawable &pEntity, OdStreamBuf &pOutStream, bool bTextMode, double dDeviation, bool positiveOctant = true);
- OdResult exportSTLEx(OdDbBaseDatabase *pDb, const OdGiDrawable &pEntity, OdStreamBuf &pOutStream, bool bTextMode, double dDeviation, bool positiveOctant = true);
The difference between the functions is that the exportSTLEx() function checks the solid topology before exporting and inverts the orientation of triangles when the signed volume of a triangulated solid is less than 0.
Note: In the original STL specification, all STL coordinates were required to be positive numbers (>= 0.01), but this restriction is no longer enforced and negative coordinates are commonly encountered in .stl files today. If you do not need to move all coordinates to a positive octant, set the last parameter to "false".
Example of exporting to an STL file using the STLExport module
This example illustrates using the STLExport module to export several solids (a torus and sphere) to an .stl file.
STLModulePtr stlModule = odrxDynamicLinker()->loadModule(OdSTLExportModuleName);
OdDb3dSolidPtr pSphere = OdDb3dSolid::createObject();
pSphere->createSphere(5);
OdGeExtents3d extents;
pSphere->getGeomExtents(extents);
double dDeviation = extents.minPoint().distanceTo(extents.maxPoint()) / 100.;
OdDb3dSolidPtr pTorus = OdDb3dSolid::createObject();
pTorus->createTorus(10, 5);
OdGeVector3d transl(15, 15, 15);
pTorus->transformBy(transl);
OdDbBlockTableRecordPtr blockTblRcrd = OdDbBlockTableRecord::createObject();
blockTblRcrd->appendOdDbEntity(pSphere);
blockTblRcrd->appendOdDbEntity(pTorus);
blockTblRcrd->setName("block1");
OdDbDatabasePtr pDb = pHostApp->createDatabase();
OdDbBlockTablePtr bt = pDb->getBlockTableId().safeOpenObject(OdDb::kForWrite);
bt->add(blockTblRcrd);
OdDbBlockReferencePtr blockRef = OdDbBlockReference::createObject();
blockRef->setBlockTableRecord(blockTblRcrd->objectId());
blockRef->setDatabaseDefaults(pDb);
OdDbBlockTableRecordPtr btr = pDb->getModelSpaceId().safeOpenObject(OdDb::kForWrite);
btr->appendOdDbEntity(blockRef);
bool asciiFormat = true; // saving as a text file, not binary
OdString fileName("sphere_torus.stl");
OdStreamBufPtr pOutStream = odrxSystemServices()->createFile(fileName, Oda::kFileWrite, Oda::kShareDenyNo, Oda::kCreateAlways);
stlModule->exportSTL(pDb, *blockRef, *pOutStream, asciiFormat, dDeviation);
The next image illustrates the export result.
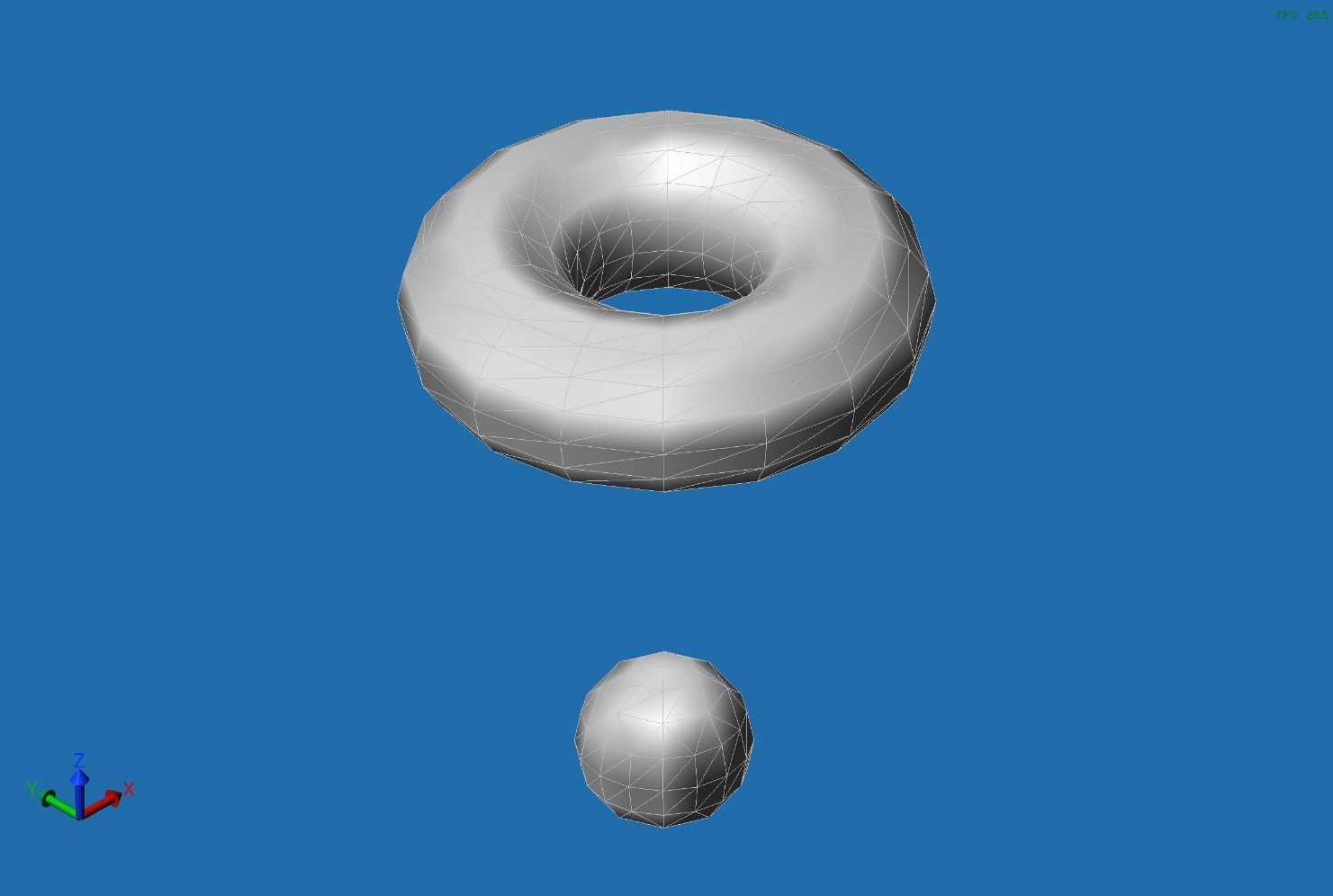
Exporting to STL using OdDb3dSolid
Use OdDb3dSolid to export only one solid to STL format. For this purpose there are two functions in the OdDb3dSolid class:
- OdResult stlOut(OdStreamBuf* output, bool asciiFormat, double maxSurfaceDeviation) const
- OdResult stlOut(const OdChar* filename, bool asciiFormat, double maxSurfaceDeviation) const
The difference between the functions is that the second function creates a file, while the first one exports to a stream.
Note: All coordinates are moved into the positive octant >= 0.01.
Example of exporting to an STL file using OdDb3dSolid
This example illustrates exporting one solid (a sphere) to an .stl file using functions of the OdDb3dSolid class:
OdDb3dSolidPtr pSphere = OdDb3dSolid::createObject();
pSphere->createSphere(5);
OdDbDatabasePtr pDb = pHostApp->createDatabase();
pSphere->setDatabaseDefaults(pDb);
OdGeExtents3d extents;
pSphere->getGeomExtents(extents);
double dDeviation = extents.minPoint().distanceTo(extents.maxPoint()) / 1000.;
bool asciiFormat = true; // saving as a text file, not binary
OdString fileName("sphere.stl");
pSphere->stlOut(fileName.c_str(), asciiFormat, dDeviation);
The next image illustrates the export result.
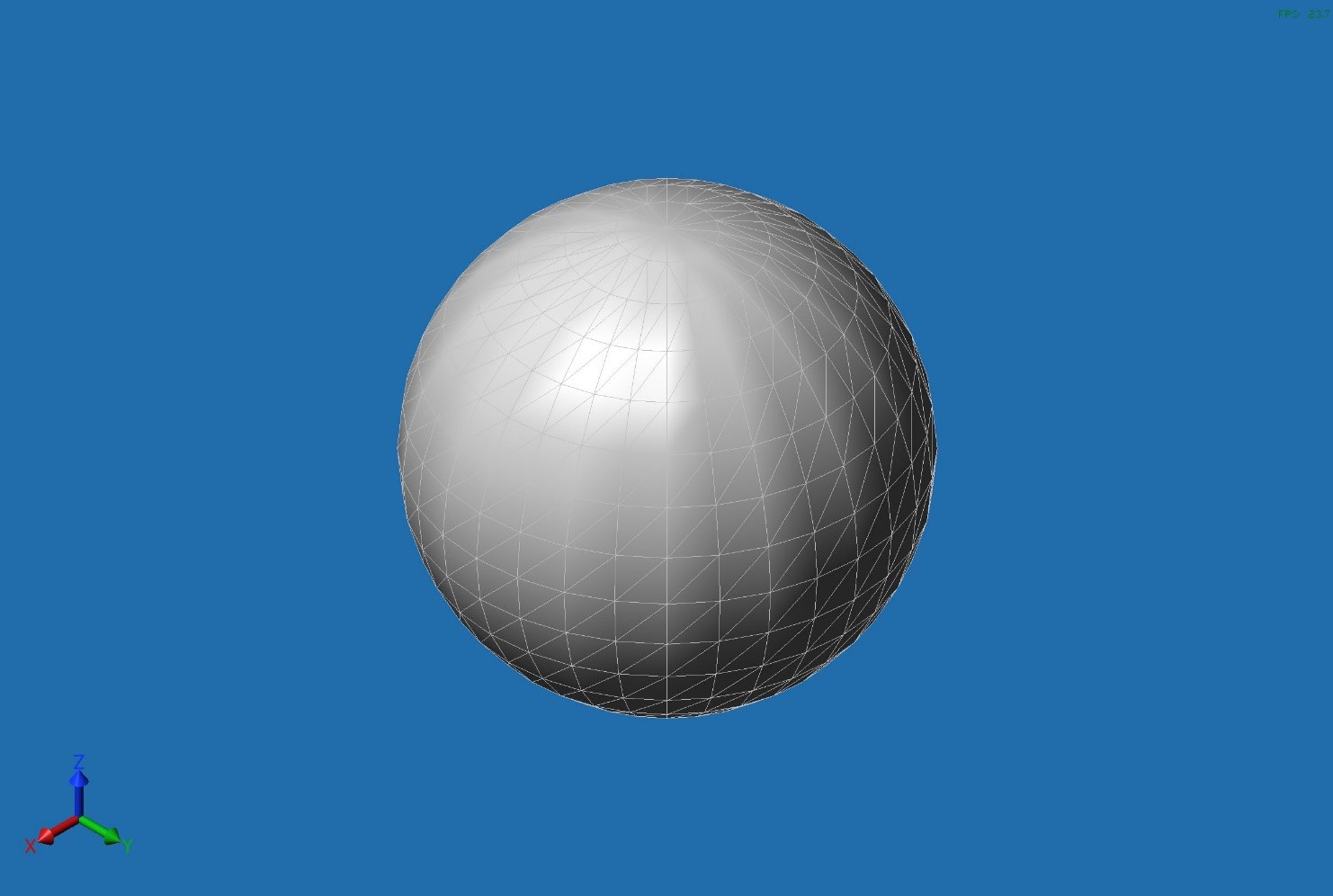