Similar to Teigha Drawings, Teigha BIM also has visual styles for rendering elements in a View. However, with Teigha Drawings visual styles are separate elements in the database, and with Teigha BIM visual styles are part of the View.
OdBmDBView has the getViewDisplayMgr() method, which returns a pointer to the View Display Manager (OdBmViewDisplayMgr). This manager allows you to set up the rendering mode of elements in the View, transparency of elements, edge visibility, and jitter effect for edges, silhouettes and shadows of elements.
Pic. 1 shows a GUI interface implemented in OdaBimApp used to set up visual styles for the active View.
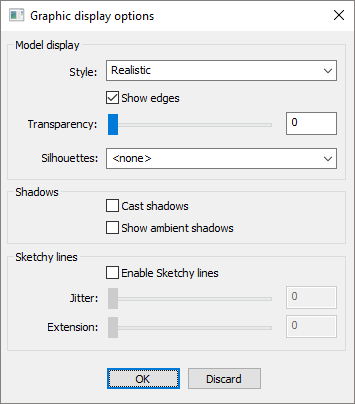
Pic. 1 Graphic display options dialog in OdaBimApp example.
Applying visual styles to elements in View
The code below is an event handler for the OK button of the dialog in Pic.1. It demonstrates how to set up rendering style properties.
void ViewDisplayStyleDialog::OnBnClickedOk()
{
m_pDBView->upgradeOpenMode();
const OdBmViewDisplayMgrPtr& pVDM = m_pDBView->getViewDisplayMgr();
OdBmViewDisplayModelPtr pModel = pVDM->getModel();
pModel->setTransparency(m_nTransparencyEditValue);
pModel->setEdges(m_bShowEdges);
switch (m_nDisplayStyle)
{
case ViewDisplayStyleDialog::kWireframe:
pModel->setDisplayStyle(OdBm::ViewDisplayStyle::Wireframe);
break;
case ViewDisplayStyleDialog::kHiddenLine:
pModel->setDisplayStyle(OdBm::ViewDisplayStyle::HiddenLine);
break;
case ViewDisplayStyleDialog::kShaded:
if (m_bShowEdges)
pModel->setDisplayStyle(OdBm::ViewDisplayStyle::ShadedWithEdges);
else
pModel->setDisplayStyle(OdBm::ViewDisplayStyle::Shaded);
break;
case ViewDisplayStyleDialog::kConsistentColors:
pModel->setDisplayStyle(OdBm::ViewDisplayStyle::ConsistentColors);
break;
case ViewDisplayStyleDialog::kRealistic:
if (m_bShowEdges)
pModel->setDisplayStyle(OdBm::ViewDisplayStyle::RealisticWithEdges);
else
pModel->setDisplayStyle(OdBm::ViewDisplayStyle::Realistic);
break;
default:
break;
}
OdBmViewDisplaySketchyLinesPtr pSketchyLines = pVDM->getSketchyLines();
if (!pSketchyLines.isNull()) {
pSketchyLines->setEnableSketchyLines(m_bSketchyLines);
pSketchyLines->setJitter(m_nJitterSliderValue);
pSketchyLines->setExtension(m_nExtensionSliderValue);
}
OdBmViewDisplayShadowsPtr pShadows = pVDM->getShadows();
if (!pShadows.isNull()) {
pShadows->setCastShadows(m_bCatShadows);
pShadows->setAmbientShadows(m_bAmbientShadows);
}
m_pDBView->downgradeOpenMode();
CString str1;
int len = m_SilhouettesStyles.GetLBTextLen(m_SilhouettesStyles.GetCurSel());
m_SilhouettesStyles.GetLBText(m_SilhouettesStyles.GetCurSel(), str1.GetBuffer(len));
std::map<OdString, OdBmObjectId>::const_iterator it =
m_SilhouetteLines.find(str1.GetBuffer());
if (it != m_SilhouetteLines.end())
pModel->setSilhouetteEdgesGStyleId(it->second);
else
pModel->setSilhouetteEdgesGStyleId(OdBmObjectId());
CDialog::OnOK();
m_nModalResult = IDOK;
}
This will change the rendering style and effect parameters, but will not apply the changes to the rendering of elements. For this, the active DbView should be set as the visual style object for the active GsView.
An empty objectId should be set to GsView to turn off visual styles for the current view.
void OdaBimExViewer::OnViewGraphicDisplayOptions() {
OdBmDBViewPtr pDBView = getActiveDbView();
ViewDisplayStyleDialog viewDisplayStyleDialog(pDBView);
OdGsView* pView = getActiveView();
viewDisplayStyleDialog.DoModal();
int modalResult = viewDisplayStyleDialog.GetModalResult();
if (modalResult == IDOK)
pView->setVisualStyle(pDBView->objectId());
else if (modalResult == IDCANCEL)
pView->setVisualStyle(OdBmObjectId());
PostMessage(WM_PAINT);
}
Rendering device support
Not all rendering devices support visual styles.
Teigha provides visual styles and rendering effects support for the WinGLESS2 device. To enable visual styles, set the ‘UseVisualStyles’ property for the device.
OdString devicePath = theApp.settings().getGsDevicePath();
if (devicePath.isEmpty()) return;
setDevicePath(devicePath);
OdGsModulePtr pGs = ::odrxDynamicLinker()->loadModule(devicePath, false);
OdGsDevicePtr pDevice = pGs->createDevice();
OdRxDictionaryPtr pProperties = pDevice->properties();
if (!pProperties.isNull())
{
...
if (pProperties->has(OD_T("UseVisualStyles"))) // Check if property is supported
pProperties->putAt(OD_T("UseVisualStyles"), OdRxVariantValue(true));
}
...
Another example of setting the visual styles up:
{
OdGsView* pView = pDevice->viewAt(0);
OdBmDBDrawingInfoPtr pDBDrawingInfo = pDb->getAppInfo(OdBm::ManagerType::DBDrawingInfo);
OdBmDBDrawingPtr pCurrDrawing = pDBDrawingInfo->getActiveDBDrawingId().safeOpenObject();
OdBmObjectIdArray viewports;
pCurrDrawing->getViewports(viewports);
OdBmViewportPtr pViewport = viewports[0].safeOpenObject();
OdBmDBViewPtr pDbView = pViewport->getDbViewId().safeOpenObject();
pDBView->upgradeOpenMode();
pDBView->setDisplayStyle(OdBm::ViewDisplayStyle::ShadedWithEdges);
pDBView->setTransparency(70);
pDBView->setShowEdges(true);
pDBView->setEnableSketchyLines(true);
pDBView->setJitter(10);
pDBView->setExtension(10);
pView->setVisualStyle(pDBView->objectId());
}
Rendering examples
The pictures below show different visual styles.
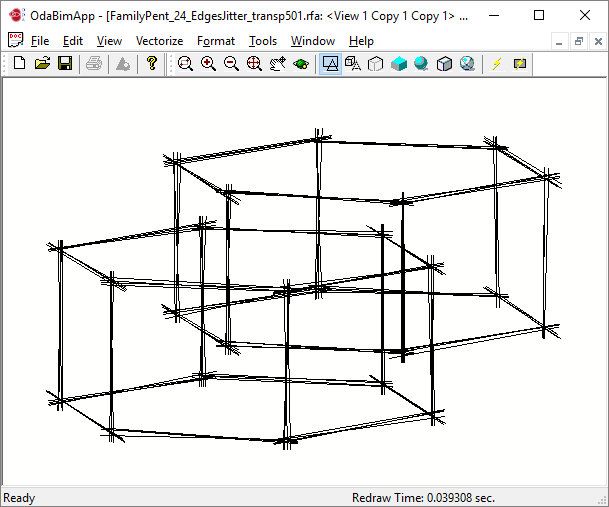
Pic. 2. Wireframe mode with jitter effect.
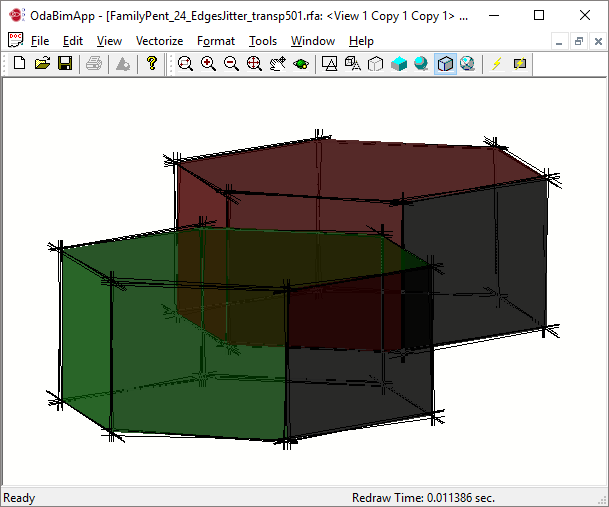
Pic. 3. Shaded mode with enabled jitter effect and 40% transparency.
Note that textures are not honored in shaded mode.
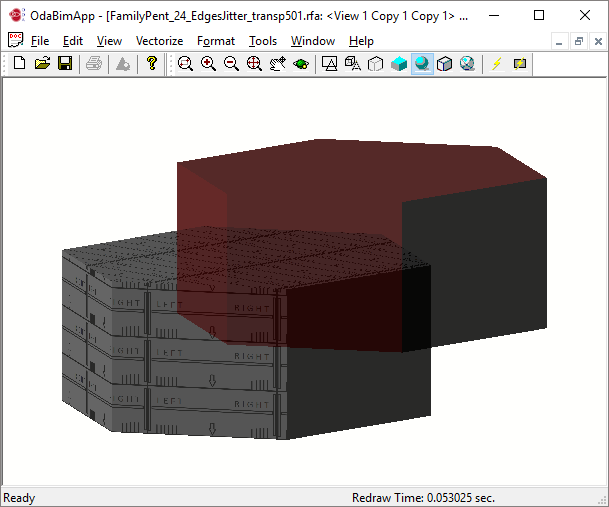
Pic. 4. Realistic mode without edges and 40% transparency.