The ODA Platform supports working with .pdf files using Google Pdfium libraries. You can convert .pdf files (or parts of the files) to raster images using the ODA SDK RxPdfToRasterSevices module, based on ODA’s TD_Pdfium module.
With the module you can implement various functionality in your applications, such as preview for .pdf export, adding .pdf attachments, and more. The RxPdfToRasterSevices module is simple to use and has flexible settings for conversion.
Let’s see how it works and convert the following .pdf file.
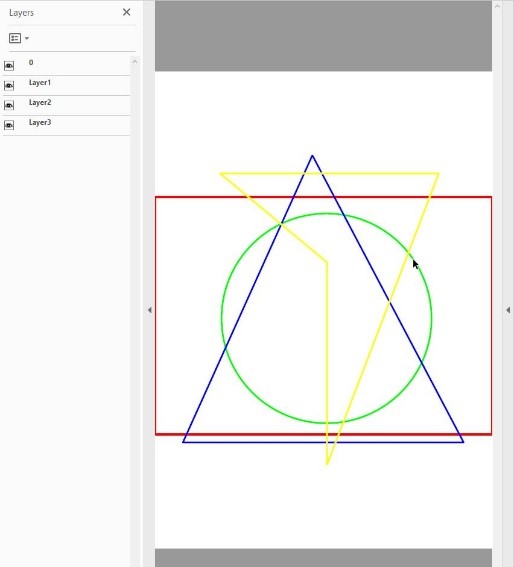
First, create the converter:
OdRxPdfToRasterServicesPtr pSvcs = odrxDynamicLinker()->loadApp(RX_PDF_TO_RASTER_SERVICES_APPNAME);
OdPdf2ImageConverterPtr pConverter = pSvcs->createConverter();
Then, load the .pdf file from disk or memory stream:
pConverter->loadPdf(L"file.pdf");
If the .pdf file contains several pages and we don’t want to convert the first one, set the active page (by default the first page is converted):
pConverter->getPagesCount();
pConverter->setActivePage(2);
If we want to convert the .pdf file as-is, this is enough, but let’s assume we want to crop the page and produce the picture with just part of the page. Then, we need to learn the page size:
OdGsDCRect rect;
pConverter->getPageSize(rect);
Now, rect contains the page size, measured in the .pdf coordinates (1/72 inches). For the current file it is 595x842. Let’s set the crop area (if this area is outside the page, the result is an empty image (a null pointer)):
OdPdf2ImageConversionParams params;
OdGsDCRect cropArea;
cropArea.m_min.x = 100;
cropArea.m_min.y = 100;
cropArea.m_max.x = 500;
cropArea.m_max.y = 500;
params.cropArea = cropArea;
We can also set the resolution and background color:
params.bg_color = 0xFFFFFFFF;
params.dpi = 300;
By default, the resolution is 72 (standard PDF resolution) and the background color is white. We can also enable or disable PDF layers if the file has them:
OdPdfLayerArray layers;
pConverter->getLayers(layers);
layers.at(2).is_on = false; //layer "Layer2" for this pdf file
params.layers = layers;
Now we just call conversion:
OdGiRasterImagePtr pImg;
pImg = pConverter->convertPdf(params);
The result is the picture below:
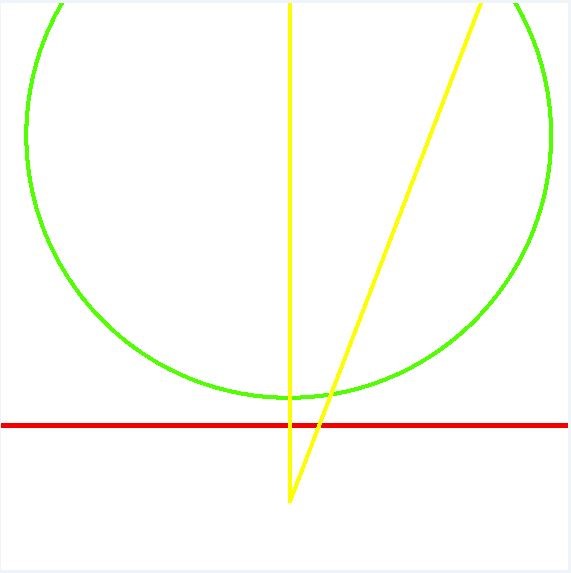
Another example is available in the ODA Drawings Debug application (OdaMfcApp.exe) in the ExportToPdfPreview dialog.
The RxPdfToRasterServices module can be used on platforms that provide C++11 support (vc14/15, gcc 4.8 and above, etc.).