You can export a portion of a large, complex drawing to PDF instead of the whole drawing. For example, you may want to export only the selected blocks that are highlighted in blue below:
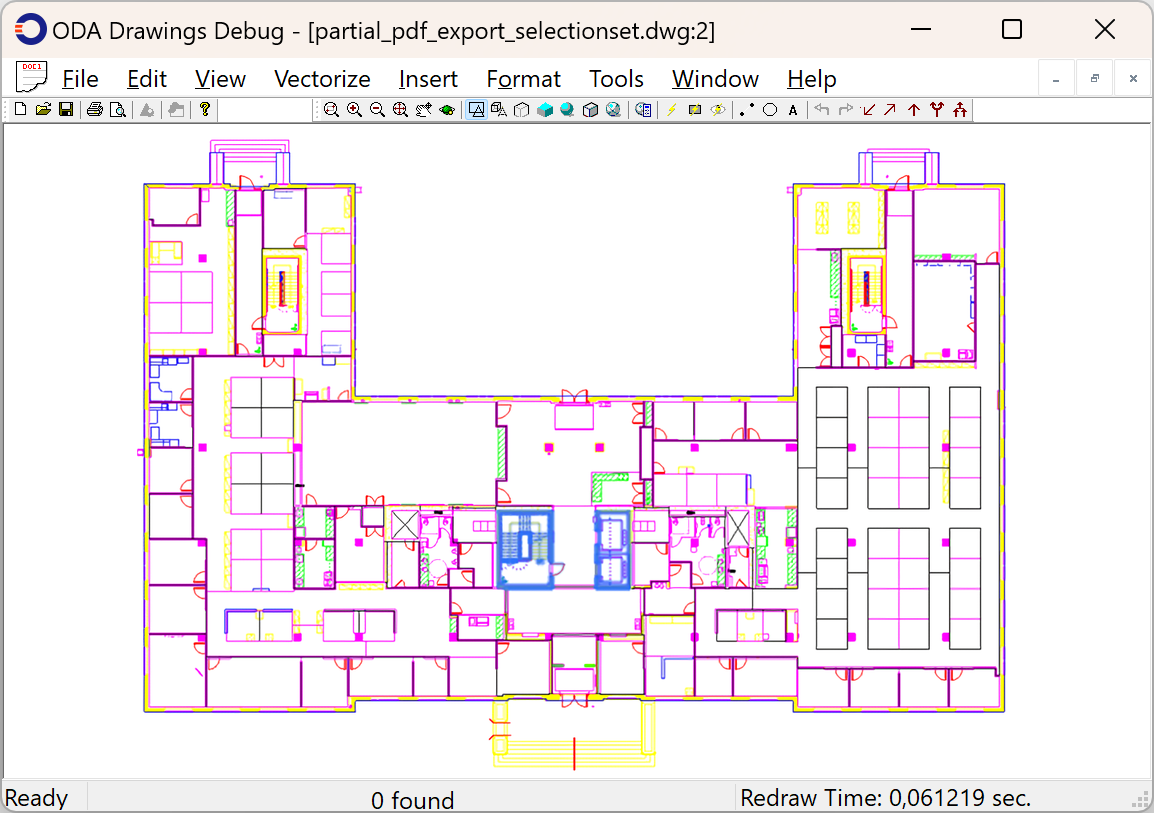
You can use the kWindow area within the PlotSettings of the drawing to export to PDF (as described in Export the Window Plot Area to PDF), but other entities or their parts may appear in the resulting PDF file if they are located in this area.
A more reliable way to export only specific entities to PDF is to use an OdDbSelectionSet object.
For example, use the following code fragments:
- Read the drawing contents in the database:
OdDbDatabasePtr pDb = pHostApp->readFile("SelectionSet.dwg");
- Set the PDF export parameters:
OdPdfExportModulePtr pPdfModule = ::odrxDynamicLinker()->loadApp(OdPdfExportModuleName); OdPdfExportPtr exporter = pPdfModule->create(); PDFExportParams params; params.setDatabase(pDb); params.setExportFlags(PDFExportParams::kZoomToExtentsMode);
- Create an empty OdSelectionSet array. Then create an OdSelectionSet object and append the identifiers of necessary entities to it. Then append the OdSelectionSet object to the array and associate the array with the PDFExportParams object.
OdArray
pSSets; OdDbSelectionSetPtr pSs = OdDbSelectionSet::createObject(pDb); pSs->append(pDb->getOdDbObjectId(OdDbHandle(0xBE))); pSs->append(pDb->getOdDbObjectId(OdDbHandle(0xAB))); pSSets.append(pSs); params.setSelectionSetsArray(pSSets);
- Export the drawing:
OdUInt32 errCode = exporter->exportPdf(params);
The output PDF document:
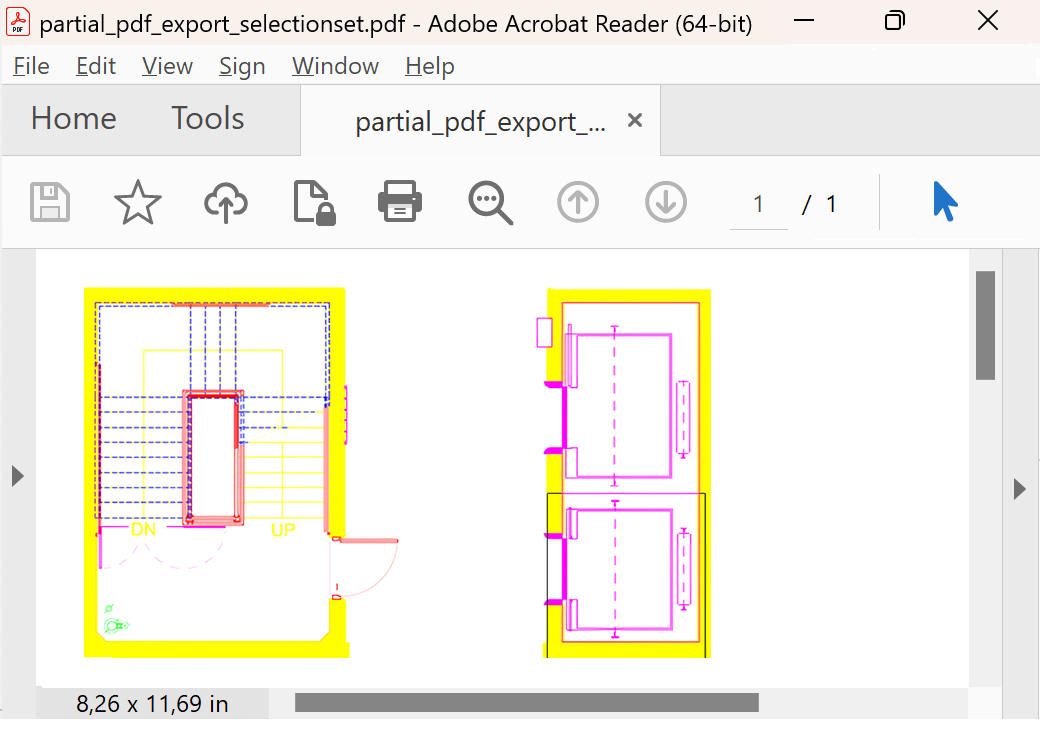
The example above illustrates exporting part of a drawing that has only one layout. Let's consider a common approach for exporting specific entities from more complicated drawings that may contain multiple layouts.
To export several layouts, create an array with the names of the layouts to be exported. Each layout is converted into a separate page in the output PDF file.
The PDFExportParams class interface provides methods that manage the list of the original drawing layouts to be exported to the PDF file:
- addLayout() — Adds a new layout name to the array of exported layouts.
- setLayouts() — Sets the string array with names of exported layouts.
- layouts() — Returns the current string array with names of exported layouts associated with the PDFExportParams object.
See Export Layouts and Set Page Parameters for PDF for additional information about exporting layouts.
After you set the array of exported layouts, you can specify the entities on the layouts that you want to export. For some layouts, you may want to export all entities. In both cases, you have to create an array of OdDbSelectionSet objects. Each element in this array corresponds to an exported layout from the drawing database and contains identifiers of layout entities that are exported to the PDF file.
The OdDbSelectionSet array must have the same size as the array of drawing layouts you want to export. To export the whole layout (all entities that belong to the layout), the corresponding OdDbSelectionSet element must be empty (the selection set doesn't contain entity identifiers).
The array of exported layouts in the PDFExportParams object must contain elements with names of all exported layouts.
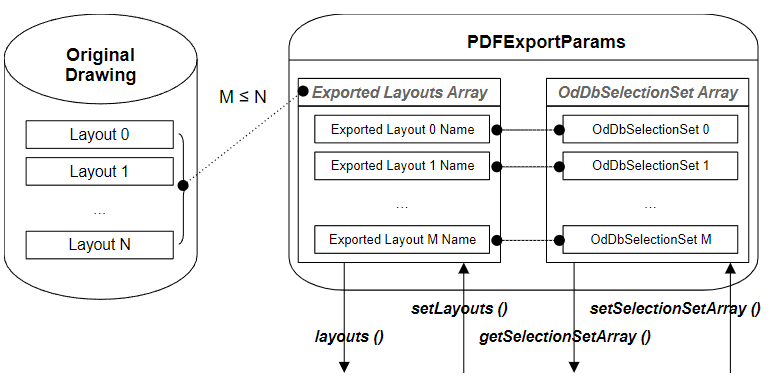
For example, a drawing has two layouts, and you want to export particular entities that belong to the first layout and all entities from the second layout. Let's assume that the array of exported layouts already contains two elements with layout names. In this case, you have to create two OdDbSelectionSet objects, although the exported entities are in the first layout only. Then you add identifiers of entities to be exported from the first layout in the first OdDbSelectionSet object and leave the second OdDbSelectionSet object empty.
To associate the OdDbSelectionSet array with a PDFExportParams class instance that handles the export parameters, call the setSelectionSetsArray() method.
There is a restriction to this approach: selected entities (or blocks) must belong to a top-level block such as model or paper space. When an entity belongs to a non-top-level block, it is not exported to PDF unless its top-level parent block is added to the OdDbSelectionSet object. And vice versa, if the top-level block is appended to the OdDbSelectionSet object, all its children entities are exported.
PRC Support for Partial PDF Export
Partial PDF export using an OdDbSelectionSet array also applies to PRC data. For example, the picture below shows a drawing with two solids:
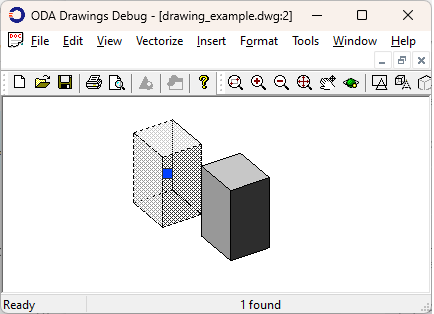
You can add the identifier of the selected solid to the OdDbSelectionSet array as described above and export only the solid entity to the 3D PDF (PRC) format:
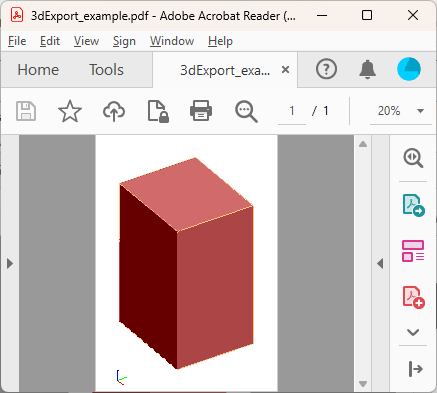
Refer to Export to PDF Using PRC for detailed information about PDF export for PRC.