You can add watermarks (plot stamps) in an exported PDF document and set different options for these watermarks.
Any object of the PDFExportParams class can contain an array of Watermark
instances, can add new watermarks to the array, and can remove all watermarks from the array. To manage watermarks, the PDFExportParams
class provides the following interface:
addWatermark()
— Adds a new watermark object to the array of watermarks.watermarks()
— Retrieves all previously added watermarks.clearWatermarks()
— Removes all watermarks from the object.
Watermark parameters are represented with the Watermark
structure which stores watermark properties:
- The text of a watermark and its attributes (font name, font size, font color, opacity). Available font names are represented with the
WatermarkFonts
enumeration.Watermark watermark; watermark.text = L"ODA Platform"; watermark.font = Watermark::kTimesRoman; //default value - Times Roman watermark.color = ODRGB(255, 0, 0); //default value – black watermark.fontSize = 24; //default value – 48 watermark.opacity = 75; //default value - 50%
Starting with the 23.3 version, the PDF Export module can use TrueType fonts installed in the operating system (see the code fragment below that illustrates the watermark parameters to set).
Watermark watermark; watermark.font = Watermark::kSystemTTFFont;//Additional enum value to keep backward compatibility watermark.fontName = L"Arial"; //Typeface
- Position on the page that is defined with the
WatermarkPosition
enumeration data type (the following pictures illustrate how a watermark looks in each position):- kLeftToRight — The watermark is located from left to right in the center of the page.
- kUpperLeft — The watermark is located in the upper-left corner of the page.
- kUpperRight — The watermark is located in the upper-right corner of the page.
- kLowerRight — The watermark is located in the lower-right corner of the page.
- kLowerLeft — The watermark is located in the lower-left corner of the page.
- kUpperLeftToLowerRight — The watermark is located from the upper-left corner to the lower-right corner of the page.
- kLowerLeftToUpperRight — The watermark is located from the lower-left corner to the upper-right corner of the page.
- kUpperMiddle — The watermark is located in the top of the page vertically and in the middle of the page horizontally.
- kLowerMiddle — The watermark is located in the bottom of the page vertically and in the middle of the page horizontally.
- kLeftMiddle — The watermark is located in the middle of the page vertically and at the left edge of the page horizontally.
- kRightMiddle — The watermark is located in the middle of the page vertically and at the right edge of the page horizontally.
The following code fragment locates the watermark in the direction from the upper-left corner to the lower-right corner of the page.
watermark.position = Watermark:: kUpperLeftToLowerRight;
- kLeftToRight — The watermark is located from left to right in the center of the page.
- Scaling option:
A watermark can also be fit to the page size by setting the corresponding flag in the
Watermark
structure (depending on the position):- Fit to the page in its center.
- Fit to the page in its upper corner (left or right).
- Fit to the page in its lower corner (left or right).
- Fit to the page in its upper-left to lower-right corner.
- Fit to the page in its lower-left to upper-right corner.
The following code fragment shows how a watermark can be fit to the page in its upper-left to lower-right corner:
watermark.color = ODRGB(255, 0, 0); watermark.opacity = 75; watermark.text = L"ODA Platform"; watermark.position = Watermark:: kUpperLeftToLowerRight; //set the scaling option: Font size in this case will be calculated automatically. watermark.scaleToPage = true;
- Fit to the page in its center.
- An offset for a watermark can be set through the offset variable of the
Watermark
structure:OdGePoint2d offset;
The offset value is represented in millimeters and its calculation depends on the watermark position (for the lower-left corner it is counted to the right and up; for the upper-right corner it is counted to the left and down, and so on).
- Rotation angle measured in radians. By setting the rotation angle value, it is possible to set vertical or upside-down text or set another angle for the watermark text:
//vertical text watermark.rotation = OdaPI2; //upside down text: watermark.rotation = OdaPI; //arbitrary watermark angle watermark.rotation = OdaToRadian(50); //the fit to page flag can also affect the rotated watermark text watermark.scaleToPage = true;
You can also apply the fit to page flag to rotated text (as in the code fragment above).
NOTE: The result of rotating watermark text may change the watermark position or even remove the watermark from the page. If necessary, the PDF Export module automatically moves the watermark to keep its position (corner or middle of the page side).
- Page index for placing a watermark on a particular page. By default, a watermark is placed on every page of the output PDF document (pageIndex property equals -1).
Watermark watermark; //adds a watermark only to the first page watermark.pageIndex = 0;
- Image as a watermark: you can add an image as a watermark instead of text. If you set an image watermark, you can't set text to the watermark (but there can be several watermarks in one document). Watermark parameters such as rotation, offset and opacity can be applied to the image watermark in the same way as a text watermark.
The following code fragment illustrates how an image can be added as a watermark with specified height and width:
Watermark watermark; watermark.scaleToPage = false; watermark.imageHeight = 50; watermark.imageWidth = 50; watermark.imagePath = pHostApp->findFile(L"pict.jpg");
Note that you can add several watermarks to the PDFExportParams
object as the following code fragment illustrates:
PDFExportParams params;
Watermark watermark;
watermark.font = Watermark::kTimesRoman; //default value - Times Roman
watermark.color = ODRGB(255, 0, 0); //default value – black
watermark.fontSize = 24; //default value – 48
watermark.opacity = 75; //default value - 50%
Set watermark text:
watermark.text = L"Watermark example 1";
watermark.position = Watermark::kUpperRight; //default - left to right in the page center
//Add the first watermark
params.addWatermark(watermark);
//Change the options of the watermark
watermark.color = ODRGB(0, 0, 255);
watermark.opacity = 50;
watermark.text = L"Scaled watermark example";
watermark.position = Watermark:: kUpperLeftToLowerRight;
//set the scaling option: Font size in this case will be calculated automatically.
watermark.scaleToPage = true;
//Add the second watermark:
params.addWatermark(watermark);
The resulting PDF file is shown in the picture below:
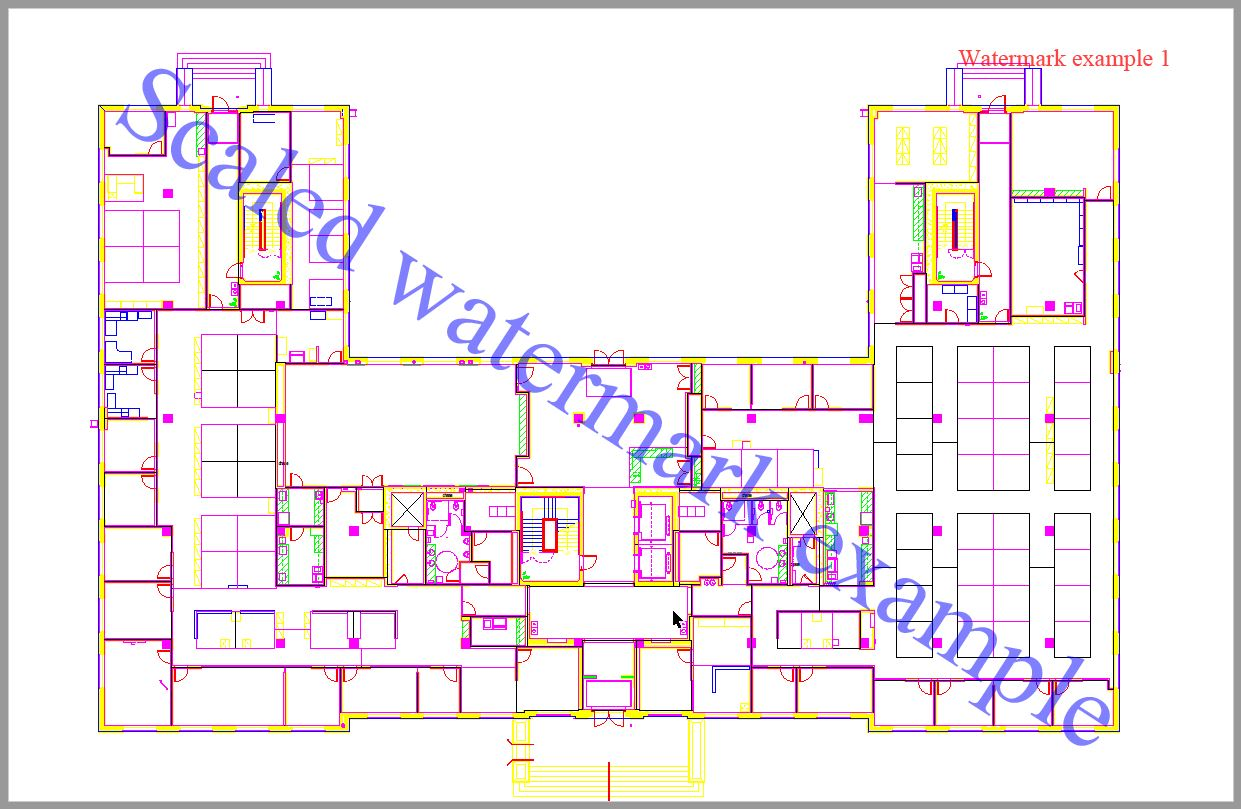
In the OdaMfcApp sample application, you can specify watermark options for PDF export using the Export to PDF dialog:
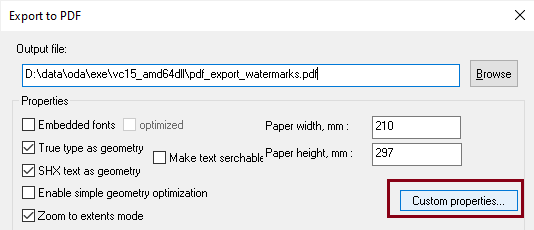
Click the Custom Properties button to open the additional Custom PDF export properties dialog, and then click the Plot stamp button to get the Plot Stamp/Watermark dialog for setting watermark options:
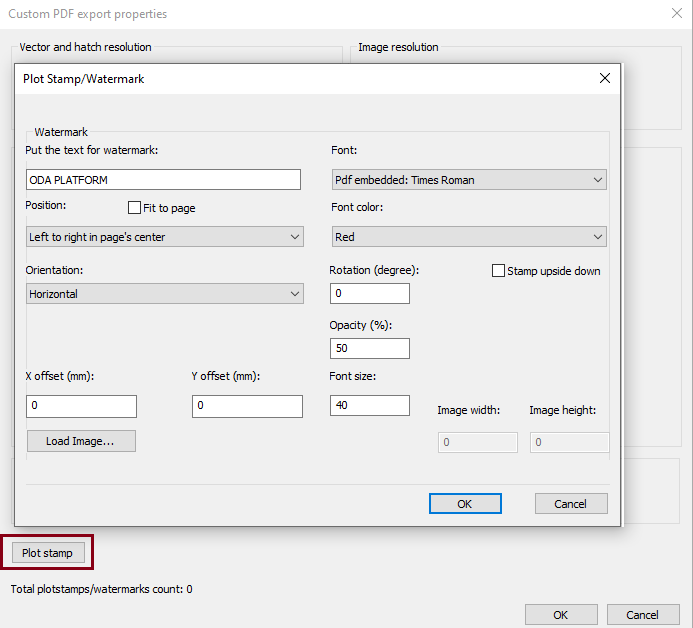
In this dialog you can specify the watermark text, text font, font size, text color, watermark position, opacity and other watermark properties.
To get more information please refer to ODA Documentation.