Overview of View Backgrounds
A view background is part of the functionality of a base Visualize Graphical View object. Visualize API provides several classes for working with view backgrounds:
- OdTvGsViewBackgroundId — Class for working with view background identifiers.
- OdTvGsViewSolidBackground - Class for working with solid view backgrounds.
- OdTvGsViewGradientBackground - Class for working with gradient view backgrounds.
- OdTvGsViewImageBackground - Class for working with image view backgrounds.
- OdTvGsViewEnvironmentBackground - Class for working with environment view backgrounds.
- OdTvGsViewBackgroundsIterator - Class for working with a view background iterator.
- OdTvGsViewBackgroundsIteratorPtr - Typified smart pointer to an instance of a solid view background iterator. Used for storing and passing references to the solid view background iterator object.
- OdTvGsViewSolidBackgroundPtr - Typified smart pointer to an instance of a solid view background. Used for storing and passing references to the solid view background object.
- OdTvGsViewGradientBackgroundPtr - Typified smart pointer to an instance of a gradient view background. Used for storing and passing references to the gradient view background object.
- OdTvGsViewEnvironmentBackgroundPtr - Typified smart pointer to an instance of an environment view background. Used for storing and passing references to the environment view background object.
- OdTvGsViewImageBackgroundPtr - Typified smart pointer to an instance of an image view background. Used for storing and passing references to the image view background object.
There are three types of a view background that can be created:
- Solid background
- Gradient background
- Image background
- Environment background
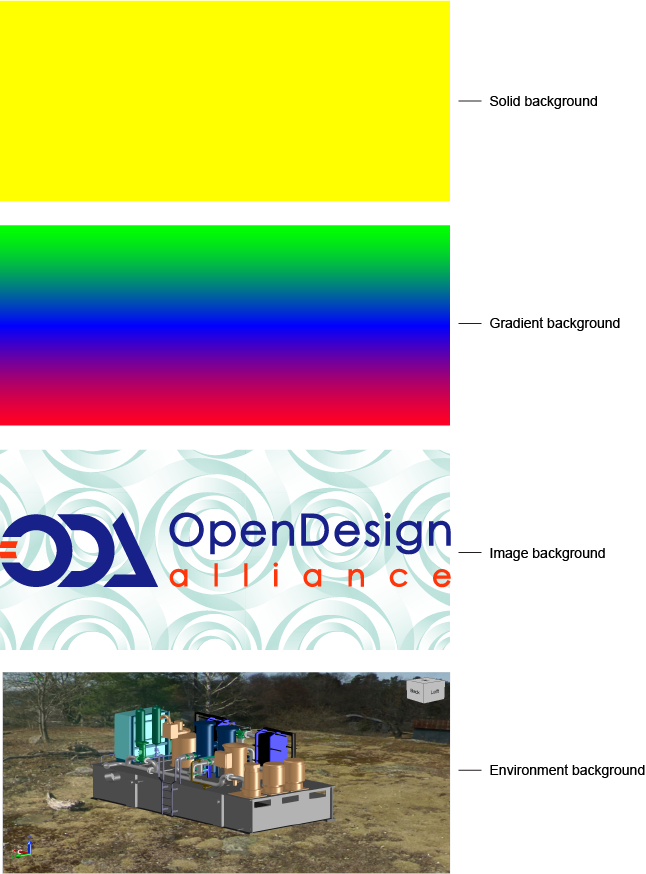
A specific view can have only one view background at a time.
To create a view background:
- Get the factory ID by using the global function odTvGetFactory:
OdTvFactoryId tvFactory = odTvGetFactory();
- Declare an OdTvResult variable that will store the result of method execution. If a method executes successfully, it sets or returns the OdTvResult value as tvOk, otherwise it contains an appropriate error code.
OdTvResult rc;
- Open or create a new Visualize SDK database using a factory object (in the code fragment a new empty database is created). Get a smart pointer to the created database:
OdTvDatabaseId databaseId = tvFactory.createDatabase(&rc); OdTvDatabasePtr dbPtr = databaseId.openObject(OdTv::kForWrite, &rc);
- Create a new device in the database. Several types of devices can be created. In this example the most simple type of device is created - a non-setup device. Get a smart pointer to the created device:
OdTvGsDeviceId deviceId0 = dbPtr->createDevice(OD_T("Device0"), &rc); OdTvGsDevicePtr pDevice0 = deviceId0.openObject(OdTv::kForWrite, &rc);
- Create a new view object. The view object is created by calling the createView() method of a device. You need to specify a view name, which must be unique in the database. The other two parameters are optional - a bool value that specifies whether a view needs to be saved in a file, and the second optional parameter accepts codes of method execution. This parameter is set to
tvOk
if the method finishes successfully. The method returns the identifier of a created view object.OdTvGsViewId viewId0 = pDevice0->createView(OD_T("View_0"), true, &rc);
- Get a smart pointer to the created view:
OdTvGsViewPtr pView0 = viewId0.openObject(OdTv::kForWrite, &rc);
- Create a view background in the database and get a smart pointer to that view background. A view background is created with the createBackground() method of the database. When creating a view background, you need to specify its name and type (a value from the OdTvGsViewBackgroundId::BackgroundTypes enumeration). Also there is one optional parameter of the OdTvResult type that holds the result of method execution. In this example a solid background is created.
OdTvGsViewBackgroundId solidBackgroundId0 = dbPtr->createBackground(OD_T("SolidBackground_0"), OdTvGsViewBackgroundId::kSolid, &rc); OdTvGsViewSolidBackgroundPtr pSolidBackground = solidBackgroundId0.openAsSolidBackground(OdTv::kForWrite, &rc);
When you get a smart pointer to a view background, you can manage its properties.
Manage View Backgrounds in a Database
Visualize API provides methods for fundamental interactions with backgrounds in a database. After at least one view background is created, you can search for background IDs by name, iterate backgrounds, remove specific backgrounds, and clear them all. The next few examples show how to perform these actions.
// Searching for background ID by its name
// if background is not found, then searchedBackgroundId.isNull() returns true
OdTvGsViewBackgroundId searchedBackgroundId = dbPtr->findBackground(OdString("SolidBackground_0"));
// Retrieving background IDs with iterator and appending to an array
OdTvGsViewBackgroundsIteratorPtr pIter = dbPtr->getBackgroundsIterator(&rc);
OdArray<OdTvGsViewBackgroundId> backgroundIdsArr;
OdTvGsDeviceId bgId;
while (!pIter->done())
{
bgId = pIter->getGsViewBackground(&rc)
if(!bgId.isNull()) // if identifier is not NULL
{
backgroundIdsArr.append(bgId);
}
pIter->step()
}
// Removing a background by the ID
rc = dbPtr->removeBackground(searchedBackgroundId);
// Clear all backgrounds
rc = dbPtr->clearBackgrounds();
Solid View Backgrounds
A solid background is a type of background that displays only one particular color. Solid backgrounds are the easiest to manage according to its color and name.
To manage the color of a solid background, use the setColorSolid() and getColorSolid() methods for setting and getting the color respectively. The default background color is black.
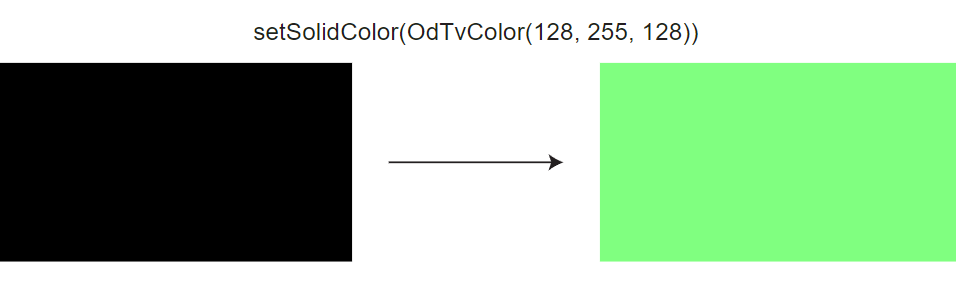
// OdTvColorDef OdTvGsViewSolidBackground::getColorSolid(OdTvResult* rc = NULL)
OdTvColorDef solidColor = pSolidBackground->getColorSolid(&rc);
// OdTvResult OdTvGsViewSolidBackground::setColorSolid(const OdTvColorDef& color)
pSolidBackground->setColorSolid(OdTvColorDef(128, 255, 128));
A name of a solid view background is an arbitrary non-empty string that can contain letters, digits, blank spaces, underscores, and some special characters, but cannot contain inadmissible letters ("<", ">", "\", "/", ":", ";", "?", ",", "*", "|", "=", "'", quotation marks and some special characters created with Unicode fonts). To set a background name use the setName() method. Use the getName() method to retrieve a string that represents a background name. This functionality is also true for other types of backgrounds.
// OdString OdTvGsViewSolidBackground::getName(OdTvResult* rc = NULL)
OdString solidBgName = pSolidBackground->getName(&rc);
// OdTvResult OdTvGsViewSolidBackground::setName(const OdString& sName)
rc = pSolidBackground->setName(OdString("SolidBG_0"));
Gradient View Backgrounds
A gradient background is a type of background that displays three colors that blend with each other. To create a gradient background, follow the instructions provided here except pass the OdTvGsViewBackgroundId::kGradient value instead of OdTvGsViewBackgroundId::kSolid to the createBackground() method in the last step.
Manage Colors of a Gradient Background
A gradient background consists of three colors — bottom, middle and top colors. By default all three colors are black. To set these colors, use corresponding set methods of the OdTvGsViewGradientBackground interface, for example the setColorBottom() method. You can also get the colors with corresponding get methods.
The next example shows how to use these methods.
// OdTvColorDef OdTvGsViewGradientBackground::getColorBottom(OdTvResult* rc = NULL) const
OdTvColorDef bottomColor = pGradientBackground->getColorBottom(&rc);
// OdTvColorDef OdTvGsViewGradientBackground::getColorMiddle(OdTvResult* rc = NULL) const
OdTvColorDef middleColor = pGradientBackground->getColorMiddle(&rc);
// OdTvColorDef OdTvGsViewGradientBackground::getColorTop(OdTvResult* rc = NULL) const
OdTvColorDef topColor = pGradientBackground->getColorTop(&rc);
// OdTvResult OdTvGsViewGradientBackground::setColorBottom(const OdTvColorDef& color)
rc = pGradientBackground->setColorBottom(OdTvColorDef(255, 0, 0));
// OdTvResult OdTvGsViewGradientBackground::setColorMiddle(const OdTvColorDef& color)
rc = pGradientBackground->setColorMiddle(OdTvColorDef(0, 0, 255));
// OdTvResult OdTvGsViewGradientBackground::setColorTop(const OdTvColorDef& color)
rc = pGradientBackground->setColorTop(OdTvColorDef(0, 255, 0));
Manage Transformational Properties of a Gradient Background
A gradient background consists of three colors as described previously. You can set the proportions of the color coverage with the setHorizon() and the setHeight() methods. Here a horizon is a double value in the range [0, 1] where 0 corresponds to 0 coordinates and 1 corresponds to the maximum coordinates for both x- and y-axes of a device window. For example, the setHorizon(0.5) method call sets a middle color to reside in the middle of the window (if no rotation is applied, which is the default).
The height parameter controls the coverage of the middle color. For example, the setHeight(0.1) method call sets a middle color to occupy 10 percent of window space (on the y-axis by default).
The other two colors are separated by the middle color and their window coverage depends on transformational properties. For example, the setHorizon(0.5) and setHeight(0.001) method calls indicate that the other two colors are distributed evenly and occupy almost all window space.
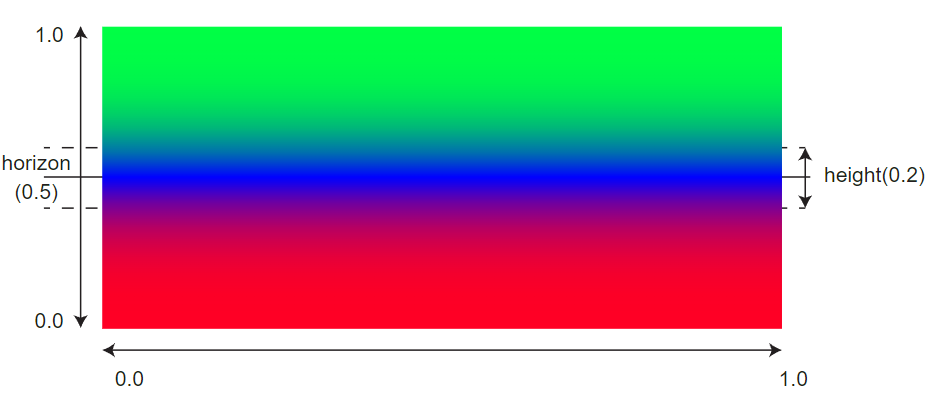
You can also rotate a background by calling the setRotation() method. This method accepts a double value that species the number of degrees of rotation. Rotation is measured in a counterclockwise direction. Note that degrees are measured not as you might expect. A 45 degree angle means that a horizon line will extend from point (0.0, 0.0) to point (1.0, 1.0), representing the beginning and end of coordinates for a device window.
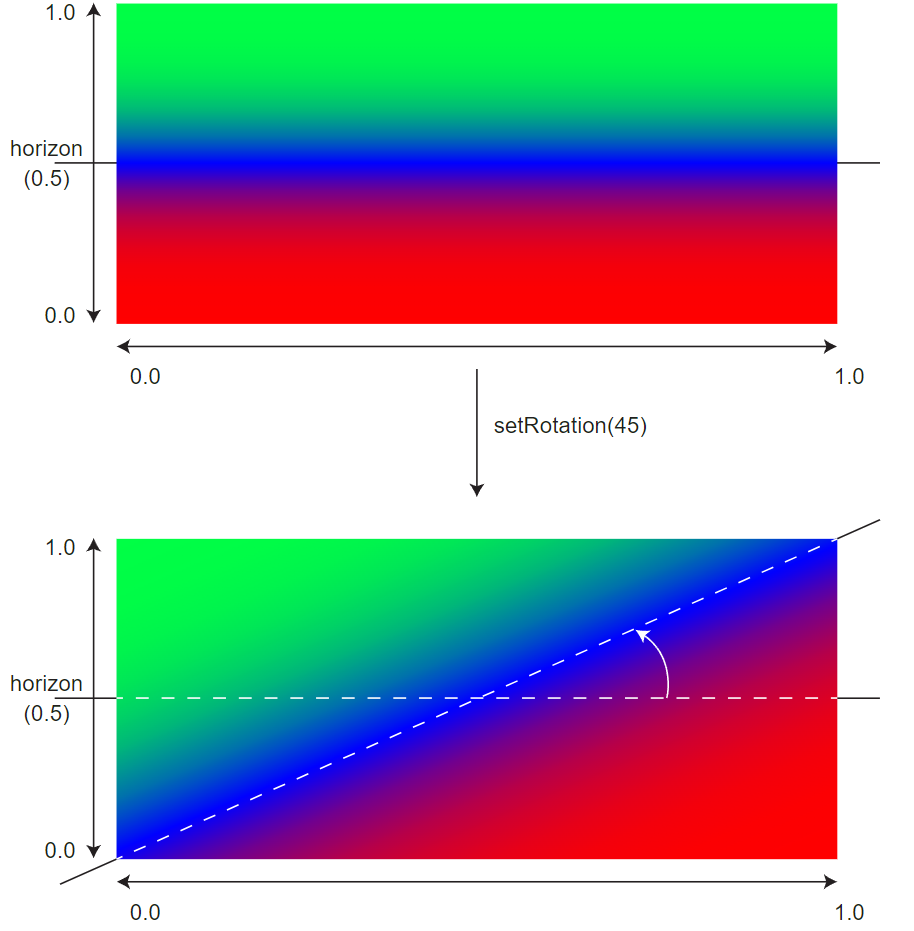
There are also get methods for getting values of transformational background properties.
The next example demonstrates how to use methods related to background transformational properties.
// double OdTvGsViewGradientBackground::getHorizon(OdTvResult* rc = NULL) const
double dHorizon = pGradientBackground->getHorizon(&rc);
// double OdTvGsViewGradientBackground::getHeight(OdTvResult* rc = NULL) const
double dHeight = pGradientBackground->getHeight(&rc);
// double OdTvGsViewGradientBackground::getRotation(OdTvResult* rc = NULL) const
double dRotation = pGradientBackground->getRotation(&rc);
// OdTvResult OdTvGsViewGradientBackground::setHorizon(double dHorizon)
rc = pGradientBackground->setHorizon(0.33);
// OdTvResult OdTvGsViewGradientBackground::setHeight(double dHeight)
rc = pGradientBackground->setHeight(0.66);
// OdTvResult OdTvGsViewGradientBackground::setRotation(double dRotation)
rc = pGradientBackground->setRotation(45.0);
Image View Backgrounds
An image background is a type of background that displays an image from a file. Image backgrounds can be useful when you need a visual reference to a kind of blueprint or drawing that you try to recreate. To create an image background, follow the instructions provided here except pass the OdTvGsViewBackgroundId::kImage value instead of OdTvGsViewBackgroundId::kSolid to the createBackground() method in the last step.
Manage an Image Path for an Image Background
To display an image background, set the file path from which an image is loaded. Use the setImageFilename() method to specify the path and file name (with extension) to load. You can also get the file path from which an image is loaded with the getImageFilename() method.
// OdTvResult OdTvGsViewImageBackground::setImageFilename(const OdString& filename)
rc = pImageBackground->setImageFilename(OdString("D://example_files//ODA2.png"));
// OdString OdTvGsViewImageBackground::getImageFilename(OdTvResult* rc = NULL) const
OdString imageFileName = pImageBackground->getImageFilename(&rc);
Fit an Image to the Screen
A background image is mapped directly to the drawing area where it is displayed. Usually images from which backgrounds are created do not fit perfectly in the device window. You can use scale-related methods, however you need to be very precise with them and usually it is difficult to make the image fit perfectly in the device window with these methods. Instead you can use the setFitToScreen() method to automatically resize the image to fit the device window. You can also use the setMaintainAspectRatio() method if you want the aspect ratio to remain unchanged when fitting to a window.
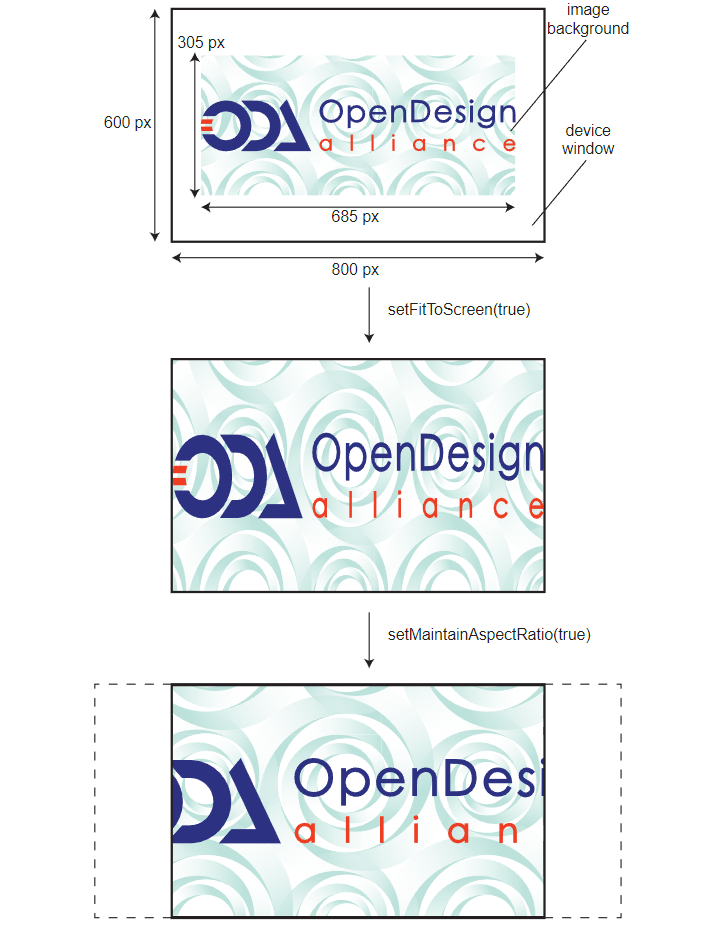
// bool OdTvGsViewImageBackground::getFitToScreen(OdTvResult* rc = NULL) const
bool bFitToScreen = pImageBackground->getFitToScreen(&rc);
// OdTvResult OdTvGsViewImageBackground::setFitToScreen(bool bFitToScreen)
rc = pImageBackground->setFitToScreen(true);
// bool OdTvGsViewImageBackground::getMaintainAspectRatio(OdTvResult* rc = NULL) const
bool bKeepAspectRatio = pImageBackground->getMaintainAspectRatio(&rc);
// OdTvResult OdTvGsViewImageBackground::setMaintainAspectRatio(bool bMaintainAspectRatio)
rc = pImageBackground->setMaintainAspectRatio(true);
When a background image occupies less space than the device window (usually when the scale is small or the image itself is small), you have an option to tile the image. This way the background repeats itself in all directions. To adjust the number of repetitions, use scale-related methods and tweak the position of copies by using offset-related methods.
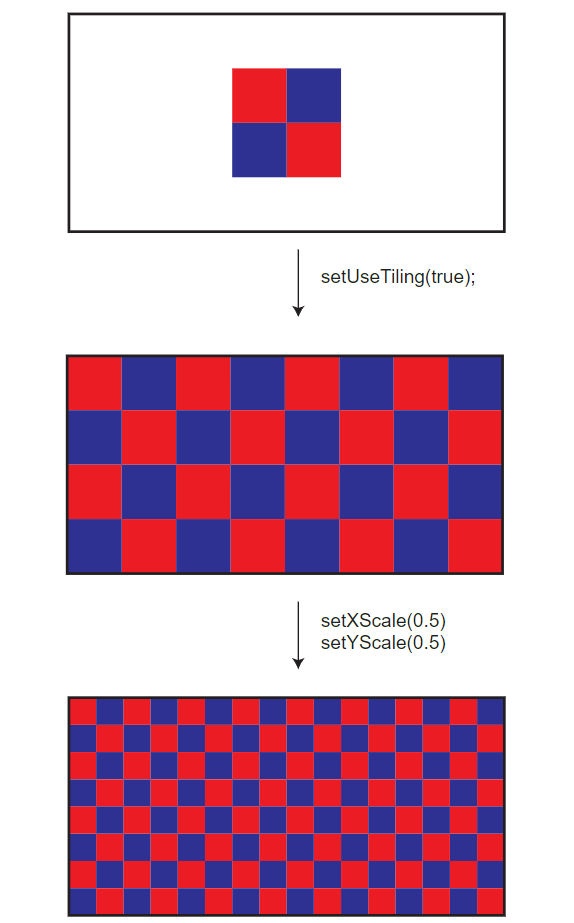
// bool OdTvGsViewImageBackground::getUseTiling(OdTvResult* rc = NULL) const
bool bUseTiling = pImageBackground->getUseTiling(&rc);
// OdTvResult OdTvGsViewImageBackground::setUseTiling(bool bUseTiling)
rc = pImageBackground->setUseTiling(true);
Manage an Image Background Offset
By default an image background is located in the center of the drawing region with an anchor point in the center of the image. An image background can be offset by x- and y-axes individually. The getXOffset() and getYOffset() methods return the number of pixels by which a background is offset. If both methods return 0.0 values, the background is centered in the drawing area. The setXOffset() and setYOffset() methods allow you to specify how much a background is offset from the center.
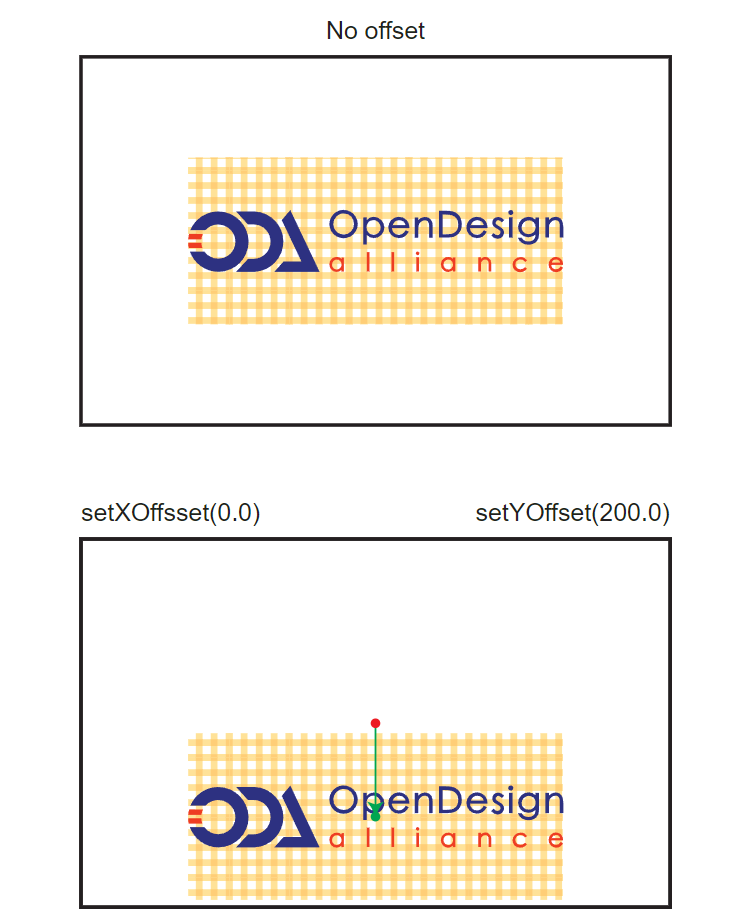
// double OdTvGsViewImageBackground::getXOffset(OdTvResult* rc = NULL) const
double dXOffset = pImageBackground->getXOffset(&rc);
// double OdTvGsViewImageBackground::getYOffset(OdTvResult* rc = NULL) const
double dYOffset = pImageBackground->getYOffset(&rc);
// OdTvResult OdTvGsViewImageBackground::setXOffset(double dXOffset)
rc = pImageBackground->setXOffset(0.0);
// OdTvResult OdTvGsViewImageBackground::setYOffset(double dYOffset)
rc = pImageBackground->setYOffset(200.0);
Manage Image Background Scales
Background images often do not fit the drawing area or vice versa — they can be too small, therefore you might need to adjust the scale of image backgrounds. To adjust the scale of an image background, use the setXScale() and setYScale() methods for the x- and y-axis respectively. To retrieve the image background scale on the x- and y-axes, use the getXScale() and getYScale() methods. An image background scale is 1.0 by default for both axes.
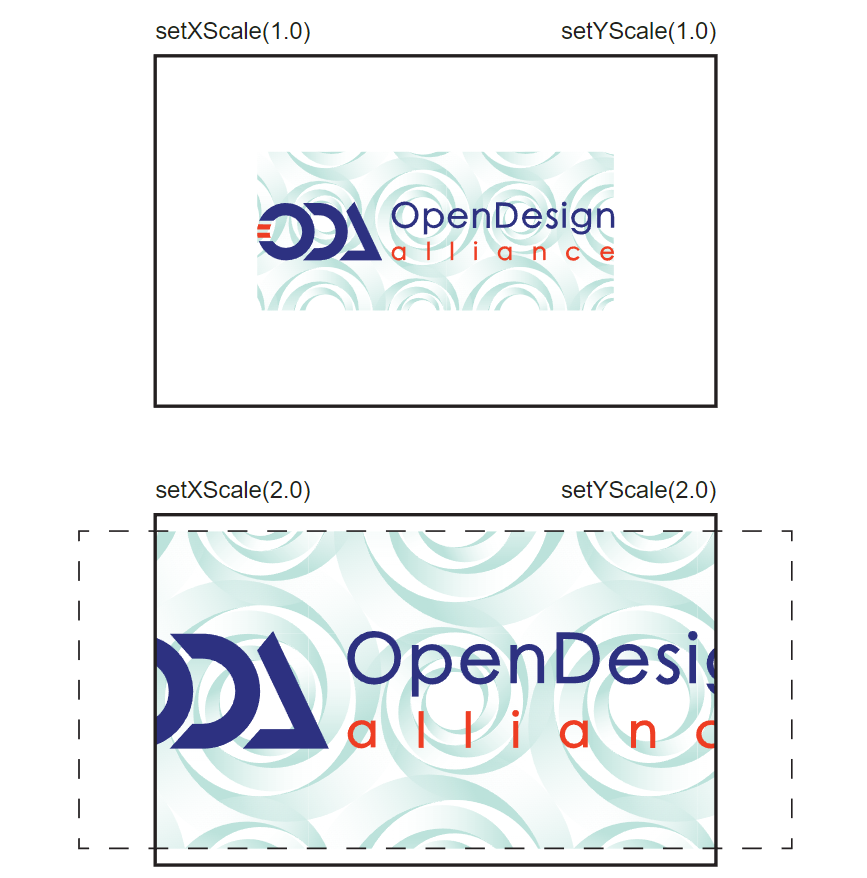
// double OdTvGsViewImageBackground::getXScale(OdTvResult* rc = NULL) const
double dXScale = pImageBackground->getXScale(&rc);
// double OdTvGsViewImageBackground::getYScale(OdTvResult* rc = NULL) const
double dYScale = pImageBackground->getYScale(&rc);
// OdTvResult OdTvGsViewImageBackground::setXScale(double dXScale)
rc = pImageBackground->setXScale(2.0);
// OdTvResult OdTvGsViewImageBackground::setYScale(double dYScale)
rc = pImageBackground->setYScale(2.0);
Environment View Backgrounds
An environment background is a type of background with a raster image on a static background and material reflections in a graphics scene. The environment can be cubic (CubeMap) or spherical (SphereMap).
You can specify a cube map using:
- A specific .ocm file (see Visualize Cube Maps for more details).
- A raster image. The raster image height must be greater than the width, and the side lengths should be dividable into equal tiles: two horizontal and three vertical. A sphere map is used only when a raster is set and these conditions are not met.
The next code shows how to create environment background, get a pointer to this object, and set up parameters. OdTvDatabasePtr pDatabase is a pointer to a Visualize database instance.
OdTvResult rc;
OdTvGsViewBackgroundId backgroundId = pDatabase->createBackground("EnvironmentBackground0", OdTvGsViewBackgroundId::kEnvironment, &rc);
OdTvGsViewEnvironmentBackgroundPtr pBackground = backgroundId.openAsEnvironmentBackground(OdTv::kForWrite);
rc = pBackground->setEnvironmentImageFileName("D:\\Files\\VIS-2717\\FjaderholmarnaSphereMap.jpg");
pView->setBackground(backgroundId);
Manage an Image Path for an Environment Background
To display an image background, set the file path from which an image is loaded. Use the OdTvGsViewEnvironmentBackground::setEnvironmentImageFileName method to specify the path and file name (with extension) to load. You can also get the file path from which an image is loaded by using the OdTvGsViewEnvironmentBackground::getEnvironmentImageFileName method.
// OdTvResult OdTvGsViewEnvironmentBackground::setEnvironmentImageFileName(const OdString& sFileName)
rc = pBackground->setEnvironmentImageFileName("D:\\Files\\VIS-2717\\FjaderholmarnaSphereMap.jpg");
// OdString OdTvGsViewEnvironmentBackground::getEnvironmentImageFileName(OdTvResult* rc = NULL) const
OdString environmentFileName = pBackground->getEnvironmentImageFileName(&rc);
When the environment image is set, the renderer can display the background.
Manage Environment Longitude and Latitude
An environment background image can be rotated about both axes. Use the OdTvGsViewEnvironmentBackground::setLongitude and OdTvGsViewEnvironmentBackground::setLatitude methods to set the background longitude and latitude. The OdTvGsViewEnvironmentBackground class also has getters to retrieve these parameters. The range for these parameters is endless, however visible results are in the range [-OdaPi .. +OdaPi], where -OdaPi is 180-degree rotation in one direction, 0 means no rotation, and +OdaPi is 180-degree rotation in the other direction. By default, longitude and latitude are set to 0.
// OdTvResult OdTvGsViewEnvironmentBackground::setLatitude(double dLatitude)
rc = pBackground->setLatitude(OdaPI2);
// OdTvResult OdTvGsViewEnvironmentBackground::setLongitude(double dLongitude)
rc = pBackground->setLongitude(OdaPI4);
Default | ![]()
|
Longitude = 0.2 rad | ![]()
|
Latitude = 0.2 rad | ![]()
|
To get more information please refer to ODA Documentation.