The use of JavaScript in the production of .pdf documents allows you to create interactive .pdf documents.
JavaScript scripts can be used to:
- Validate entered data
- Perform calculations
- Dynamically change the presentation of a document
- Dynamically create and modify document objects
Description of functions
Teigha Publish SDK contains the following functions for working with JavaScript.
Functions of the class OdDocument
void addJavaScript(const OdString& name, const OdString& source);
Used to add a named JavaScript document-level script.
void getJavaScripts(OdStringArray& names, OdStringArray& sources) const;
Used to retrieve all named JavaScript document-level scripts.
The document level script is executed once when the document is opened.
Functions of the class OdPage
void addJavaScriptActionByField(const OdString& field_name, const OdString& source, const Action::Type action_type);
Used to add a JavaScript script to the unique name of the interactive form (field) that is executed in the case of user actions described by the action_type parameter. When you add a script for a custom action with an existing JavaScript script, the last added script for this action will be used.
void getJavaScriptActionsByField(const OdString& field_name, OdStringArray& sources, OdActionTypeArray& action_types) const;
Used to retrieve all JavaScript scripts assigned to an interactive form with the given name.
Interactive forms (fields) are the following Teigha Publish SDK objects: OdTextField, OdButton, OdListBox, OdCheckBox, OdDropDownList, OdRadioButton, and OdSignatureField. User-defined object data names must be unique within the same document. For each field, it is possible to respond to the following user actions:
Action :: kCursorEnter Action :: kCursorExitAction :: kButtonPressed field Action :: kButtonReleased Action :: kInputFocus Action :: kLoseFocus Action :: kPageOpened Action :: kPageClosed When the page containing the field becomes visible Action :: kPageVisible When the page containing the field is no longer displayed Action :: kPageInvisible
User Action | action_type |
Moving the cursor to the area above the interactive form |
Action :: kCursorEnter |
Moving the cursor outside the area of the interactive form |
Action :: kCursorExit |
Clicking the left mouse button inside the active field area |
Action :: kButtonPressed field |
Releasing the left mouse button inside the active field area |
Action :: kButtonReleased |
Getting the input focus field |
Action :: kInputFocus |
Losing the input focus field |
Action :: kLoseFocus |
Opening a page that contains this field |
Action :: kPageOpened |
Closing the page containing this field |
Action :: kPageClosed |
When the page containing the field becomes visible |
Action :: kPageVisible |
When the page containing the field is no longer displayed |
Action :: kPageInvisible |
Functions of class OdLink
void setJavaScript(const OdString& source);
void getJavaScript(OdString& source) const;
Used respectively for setting and retrieving a JavaScript script for this instance of the OdLink class. This script is executed when the left mouse button is released inside the active field area.
OdArtwork functions
void setJavaScript(const OdString& source);
void getJavaScript(OdString& source) const;
Used respectively for setting and retrieving a JavaScript script for this instance of the OdArtwork class. The script is executed when this object is initialized.
Example of using JavaScript scripts
Consider the use of JavaScript scripts for example objects OdButton and OdTextField.
OdFilePtr pPublisher = OdFile::createObject();
OdDocumentPtr pDoc = OdDocument::createObject();
pDoc->setInformation(L"Test Document", L"Author", L"Test", L"Oda Pdf Publish");
pDoc->setHostServices(pHostApp);
OdPagePtr pPage = OdPage::createObject();
pPage->setFormat(Page::kA3);
pPage->setOrientation(Page::kLandscape);
pDoc->addPage(pPage);
OdUInt32 top_line_top = 830;
OdUInt32 top_line_text_top = 650;
OdRect button_rect(20, 200, top_line_top - 80, top_line_top);
OdButtonPtr pButton = OdButton::createObject();
OdString button_name(L"TestButton");
pButton->setName(button_name);
pButton->setLabel(L"Test");
pPage->addButton(pButton, button_rect);
OdRect tx_button_rect(20, 200, top_line_text_top - 30, top_line_text_top);
OdTextFieldPtr tx2button = OdTextField::createObject();
OdString tx2button_name(L"ButtonText");
tx2button->setName(tx2button_name);
pPage->addTextField(tx2button, tx_button_rect);
OdChar* buttonActionBase =
L"var text_field = getField('%s');\n"
L"if(text_field != null) \n"
L" text_field.value = '%s'; \n";
pPage->addJavaScriptActionByField(button_name, OdString().format(buttonActionBase, tx2button_name.c_str(), L"button is pressed"), Action::kButtonPressed);
pPage->addJavaScriptActionByField(button_name, OdString().format(buttonActionBase, tx2button_name.c_str(), L"button is released"), Action::kButtonReleased);
pPage->addJavaScriptActionByField(button_name, OdString().format(buttonActionBase, tx2button_name.c_str(), L"cursor enters"), Action::kCursorEnter);
pPage->addJavaScriptActionByField(button_name, OdString().format(buttonActionBase, tx2button_name.c_str(), L"cursor exits"), Action::kCursorExit);
pPublisher->exportPdf(pDoc, sOutPdf);
In the code for this example, we create one OdButton object and one OdTextField object on the page. Then we add the OdButton object to several scenarios that output information about the event to the OdTextField object. The result of this code will be a .pdf document containing two controls: a button and a text field. When you move the cursor to the active area of the button or when you press / release the left mouse button in the active area of the button, a text message is displayed in the text field. The picture below shows the initial state of objects and their state as a result of the execution of user actions.
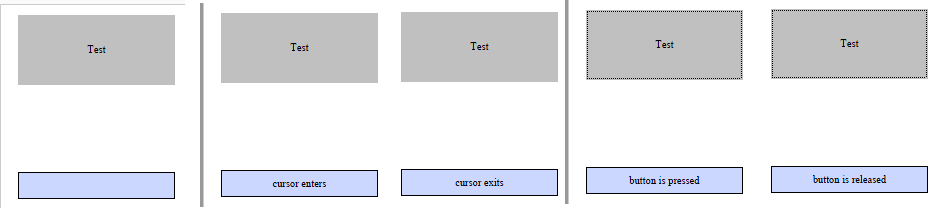