There are several ways to calculate intersections of curves and surfaces using the Ge library in ODA Kernel SDK. The most common way is to use the intersection classes derived from OdGeEntity3d:
- OdGeCurveCurveInt2d for 2D curve intersections
- OdGeCurveCurveInt3d for 3D curve intersections
- OdGeCurveSurfInt for curve-surface intersections
- OdGeSurfSurfInt for surface intersections
Another way is to use the intersectWith methods that are available for some curves and surfaces. See the API reference online (login required) for the list of all methods and their usage.
Work with Intersection Classes
Intersection classes require two input entities that can be set in a constructor or by using a set method. Intersection classes do not own their base curves and surfaces. For curve intersections, you can optionally specify the range of curves to find intersections within; in this case the domain of curves is ignored.
All intersections are calculated automatically on the first call of one of the query functions, e.g., numIntPoints. Using the set method invalidates all previously calculated intersections.
There are two types of intersections:
- Intersection points
- Intersection curves
Each intersection has its own index. Note that points and curves have an independent numeration for curve intersectors, i.e. OdGeCurveCurveInt2d and OdGeCurveCurveInt3d, but they have a shared numeration for OdGeCurveSurfInt and OdGeSurfSurfInt (see examples and images). Ends of the overlapping intersections are not returned as separate intersection points. Intersection curves can touch each other at the ends.
For OdGeCurveSurfInt and OdGeSurfSurfInt, 2D and 3D curves returned by the methods intParamCurve and intCurve are created with the operator new, and it is the responsibility of the caller to delete them. These functions return NULL if they are called twice for the same intersection.
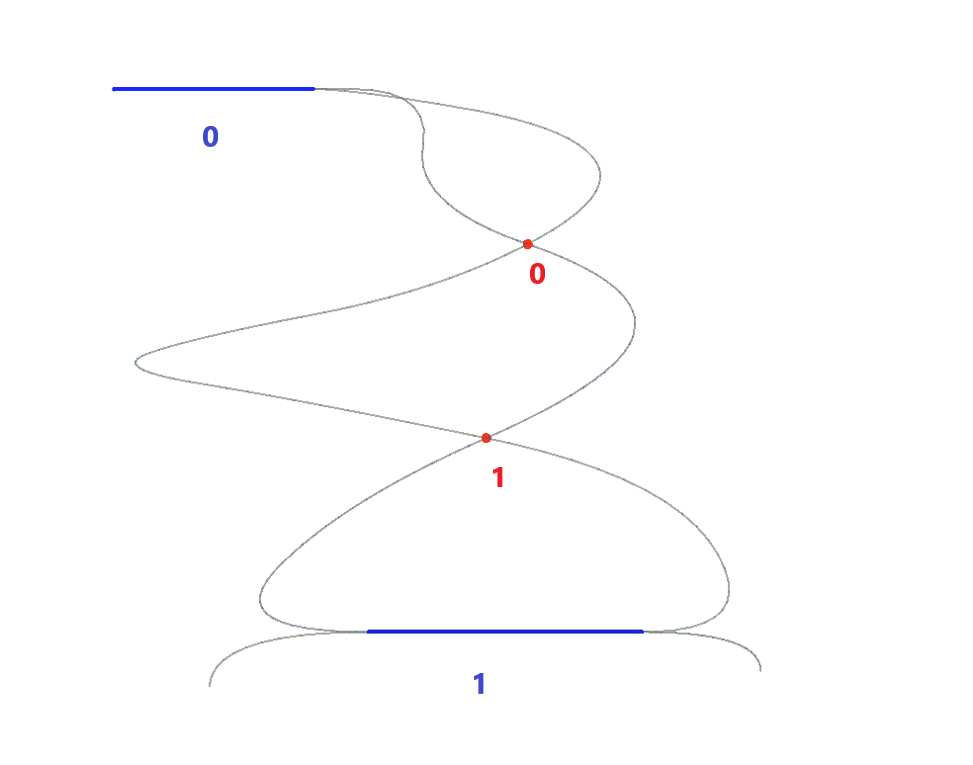
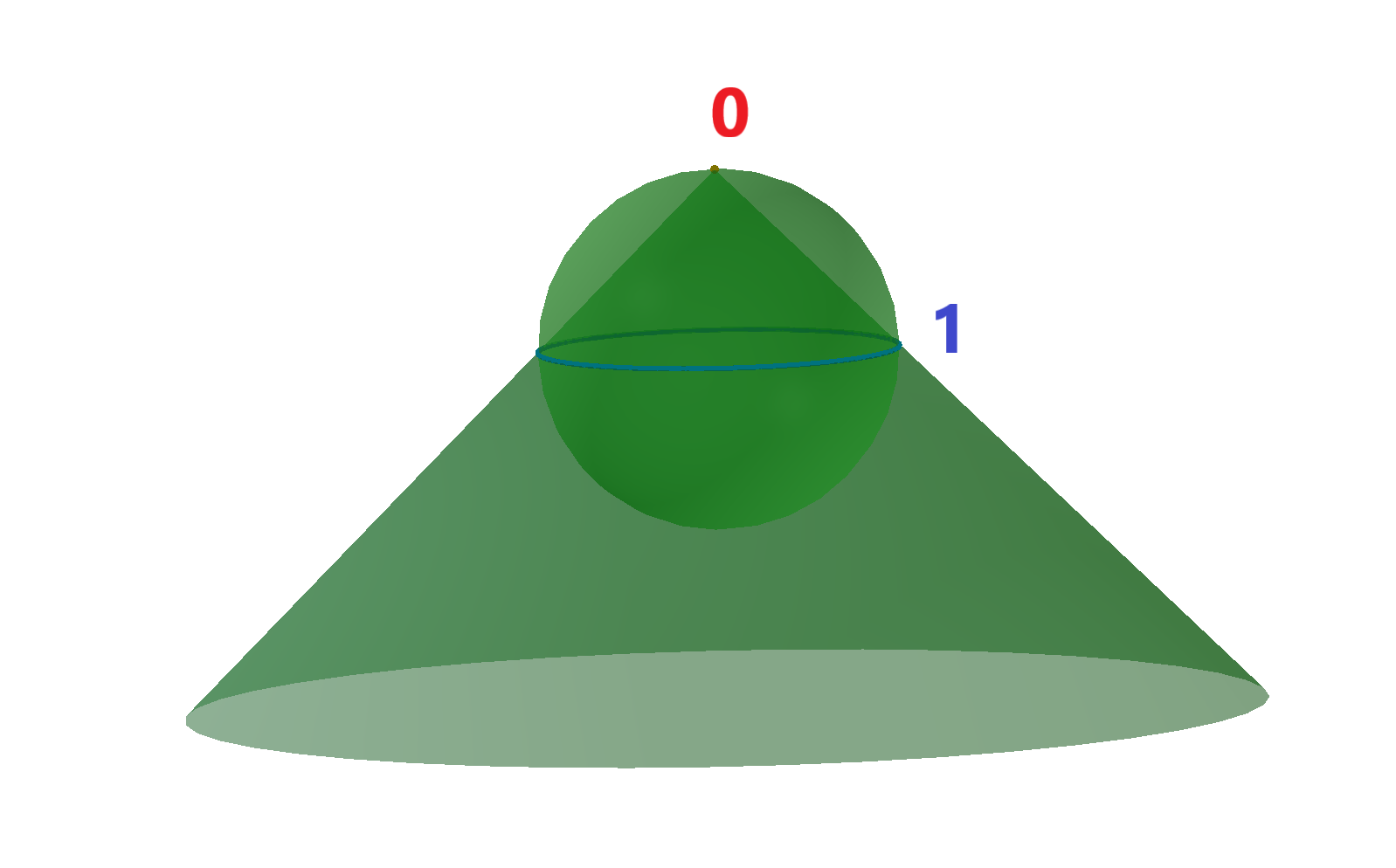
Examples:
1. OdGeCurveCurveInt2d intersector(curve1, curve2, 1e-10); //init intersector
for (int i = 0; i < intersector.numIntPoints(); ++i) //cycle for all intersection points
doSmth(intersector.intPoint(i)); //get i-th intersection point
for (int j = 0; j < intersector.overlapCount(); ++j) //cycle for all intersection curves, i.e. overlaps of the input curves
{
OdGeInterval range1, range2;
intersector.getOverlapRanges(j, range1, range2) //get ranges of the input curves, where j-th overlap is present
doSmth(range1, range2);
}
2. OdGeSurfSurfInt intersector(surf1, surf2, 1e-10); //init intersector
for (int i = 0; i < intersector.numResults(); ++i) //cycle for all intersections
{
OdGeIntersectionError status;
if (intersector.getDimension(i, status) == 0) //get dimension of the i-th intersection: point – 0, curve – 1
{
double param1, param2;
intersector.getIntParams(i, param1, param2, status); //get parameters of i-th intersection point
doSmth(param1, param2);
}
else
{
OdGeCurve3d* curve3d = intersector.intCurve(i, false, false, status); //get i-th intersection 3d curve
OdGeCurve2d* curve2d = intersector.intParamCurve(i, false, true/*false*/, status); //get i-th intersection 2d curve on first (second) input surface
doSmth(curve3d, curve2d);
delete curve3d;
delete curve2d;
}
}
Work with intersectWith Methods
For the most common intersections, there are intersectWith methods. For curve-curve or curve-surface intersections, intersectWith returns true if this entity and the parameter entity have at least one intersection point (intersection curves are ignored). The number of intersections is received in the parameter numInt. Intersection points are received in the parameters p, p1, p2, p3, and p4. Note that the intersectWith methods for linear entities retrieve an intersection point even if it lies on the continuation of entities and the returned value is false. For surface-surface intersections, intersectWith returns true if this entity and the parameter entity have an intersection curve, which is received in the parameter intLine.
Below is a list of entities that can be intersected using the intersectWith method:
- OdGeCircArc2d with
- OdGeCircArc2d
- OdGeLinearEnt2d
- OdGeEllipArc2d with
- OdGeLinearEnt2d
- OdGeLinearEnt2d with
- OdGeLinearEnt2d
- OdGeNurbCurve2d with
- OdGeLine2d
- OdGeCircArc3d with
- OdGeCircArc3d
- OdGeLinearEnt3d
- OdGePlanarEnt
- OdGeEllipArc3d with
- OdGeLinearEnt3d
- OdGePlanarEnt
- OdGeLinearEnt3d with
- OdGeLinearEnt3d
- OdGePlane3d
- OdGeBoundedPlane with
- OdGePlane
- OdGeBoundedPlane
- OdGePlane with
- OdGePlane
- OdGeBoundedPlane
- OdGeCone with
- OdGeLinearEnt3d
- OdGeCylinder with
- OdGeLinearEnt3d
- OdGeEllipCylinder with
- OdGeLinearEnt3d
- OdGeSphere with
- OdGeLinearEnt3d
- OdGeTorus with
Examples:
1. int numInt;
OdGePoint3d p1, p2;
bool intersect = circle.intersectWith(line, numInt, p1, p2, 1e-10);
if (numInt == 0)
doSmth();
if (numInt == 1)
doSmth(p1);
if (numInt == 2)
doSmth(p1, p2);
2. OdGeLineSeg3d line;
if (plane1.intersectWith(plane2, line, 1e-10))
doSmth(line);