Thanks to shadow capabilities added to ODA Kernel, you can compute and draw shadows for light sources inside a scene. This series of topics describes how to enable shadows for existing .dwg files and how shadow rendering can be configured to achieve the best results.
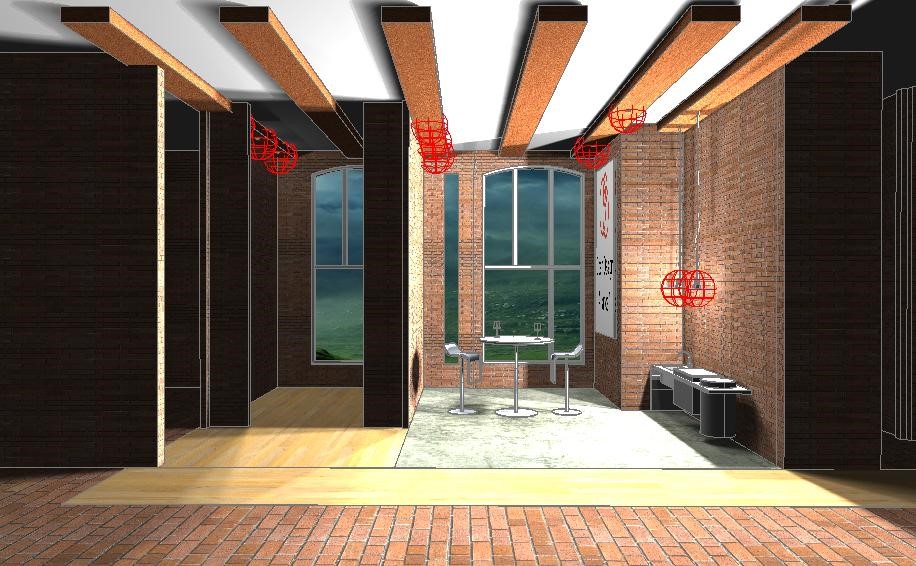
Shadows can add additional realism to existing graphics scenes if they contain light sources. They can be rendered if lighting is enabled (for shaded and realistic visual styles). Shadows are represented by the OdGiShadowParameters class and managed as a part of light parameters.
Enable Shadows for Light Sources
First, enable shadows for light sources that cast shadows inside a scene:
void configureLightSourceShadow(OdDbLight *pLight)
{
OdGiShadowParameters shadowParams = pLight->shadowParameters();
shadowParams.setShadowsOn(true);
shadowParams.setShadowType(OdGiShadowParameters::kShadowMaps);
shadowParams.setShadowMapSize(256);
shadowParams.setShadowMapSoftness(1);
pLight->setShadowParameters(shadowParams);
}
Each light source can have its own shadow parameters. Light sources are represented in an OdDbDatabase as OdDbLight entities.
Shadow parameters contain the following set methods for main properties:
- The setShadowsOn() method can be called to enable or disable shadows for a configured light source.
- The setShadowType() method can be called to set the type of shadow. GL2 Renderer always invokes shadow maps and doesn't support other modes, so it is recommended to always set this parameter as kShadowMaps.
- The setShadowMapSize() method can be called to set the size of the shadow map texture. Larger texture sizes increase shadow details but require additional resources and make final shadows crisper. Smaller texture sizes decrease shadow details but make final shadows smoother.
- The setShadowMapSoftness() method can be called to set the shadow smoothness. Currently four smoothing stages are supported:
0 | Disable smoothing. |
1 | Invoke smoothing using 3x3 Gaussian blur kernel. |
2 | Invoke smoothing using 5x5 Gaussian blur kernel. |
≥3 | Invoke smoothing using 7x7 Gaussian blur kernel. |
Since blur is executed on the GPU side, there is no serious performance difference between Gaussian kernel sizes; primarily this parameter affects the amount of blur effect (size of the blurred area).
Enable Shadows for Visual Styles
Each visual style with enabled Phong model lighting can be used to display shadows, but not all default visual styles contain the shadow property enabled by default.
void enableVisualStyleShadows(OdDbVisualStyle *pVisualStyle)
{
pVisualStyle->setTrait(OdGiVisualStyleProperties::kDisplayShadowType, (OdInt32)OdGiVisualStyleProperties::kShadowsFull);
}
To enable visual style shadows, set up the kDisplayShadowType property to something different than kShadowsNone. The kShadowsFull setting is recommended. Also it is recommended to enable per-pixel lighting, since per-face and per-vertex isn't enough to display all shadow map details.
Default Viewport Lighting
Initially, the default viewport lighting is used which you need to disable:
void defaultDefaultLights(OdDbViewport *pViewport)
{
pViewport->setDefaultLightingOn(false);
}
If default viewport lighting is enabled, the renderer draws it instead of scene lights, and as a result you cannot see any shadows from the scene lights.
The next topic in this series will describe how to work with shadows on a per-entity basis and how shadows are affected by different light types and properties.