Bill Of Materials (BOM) is a table that holds all parts and components with corresponding data and attributes. The logical representation is a table with rows, and each row refers to a part or component.
There are three ways that a BOM table can be created:
- MAIN BOM table — BOM table for all of model space.
- Assembly BOM table — BOM table for a component that holds child components inside itself.
- Border BOM table — BOM table for a title border, block reference.
Create a BOM table signature for assembly and MAIN BOMs:
OdResult createBomTable(OdDbObjectId& aNewBomTableId, const OdDbObjectId targetId, const OdString& name, bool addToBrowse, OdDbDatabase * pHostDb);
Creating a MAIN BOM
In most cases, a MAIN BOM is best for general purposes. You can create it with the BOM Manager, which is a service that allows you to work with parts and components and create BOM tables.
First, create a BOM manager service class:
AcmBOMManagerPtr pBomMng = getAcmBomMgr();
Then call the AcmBOMManager::createBomTable method with the following parameters:
- aNewBomTableId — OdDbObjectId for the new BOM.
- targetId — OdDbObjectId for the target object. For a MAIN BOM, pass empty Id — OdDbObjectId::kNull.
- Name — OdString object with the BOM table name (“MAIN” is fine).
- pDb — Pointer to the database.
OdDbObjectId newBomTbl;
pBomMng->createBomTable(newBomTbl, OdDbObjectId::kNull, L"MAIN", true, pDb);
Creating an assembly BOM
An assembly is a special component that holds other (folded) components. In this case, a BOM for an assembly also can be created with the AcmBOMManager::createBomTable method but with other parameters. Instead of OdDbObjectId::kNull (for a MAIN BOM), a component definition object should be passed as the targetId parameter.
Get a component definition:
OdDbObjectId compDefObjectId = pDb->getOdDbObjectId(compDefHandle);
Then call AcmBOMManager with parameters and with a component definition objectId as the targetId:
pBomMng->createBomTable(newBomTbl, compDefObjectId, L"MAIN", true, pDb);
Creating a border BOM
A title border is a border that defines the borders of a drawing. The title has a physical representation in the database as a BlockReference that refers to a BlockTableRecord. The title border can have its own Bill Of Materials table. A border BOM table has a special feature: it creates BOM entries (BOM rows) only for components that are located inside the title border. In this case, a BOM for a border can be created with AcmBOMManager::createBorderBomTable and with the following parameters:
OdResult createBorderBomTable(OdDbObjectId& aNewBomTableId, const OdDbObjectId borderId, OdString name = "", bool populate = false);
- aNewBomTableId — OdDbObjectId for the new BOM.
- targetId — OdDbObjectId for the target blockReference.
- Name — OdString object with the BOM table name (if empty, it will be named by default).
- populate — bool flag that allows creation of BOM rows for the BOM.
Get a blockReference:
OdDbObjectId borderId = pDb->getOdDbObjectId(borderHandle);
Then call AcmBOMManager with parameters and with a blockReference objectId as the targetId:
OdDbObjectId newBomTbl;
pBomMng->createBorderBomTable(newBomTbl, borderId, "", true);
Database changes when creating a BOM
After creating a BOM, a few new objects are added to the database. In AcmDictionary, folded AcmBomRow objects are added to the dictionary “AcmBOM” AcmBom object.
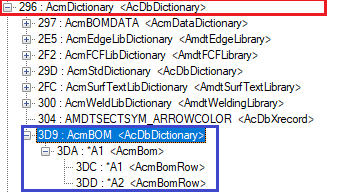
AcmDictionary state