Introduction
Starting with intermediate version 20.2, ODA SDKs contain reorganized visualization modules.
The new visualization module structure improves flexibility of ODA SDKs and increases the number of opportunities during application development. For example, you can build an application that doesn’t contain a database but requires rendering, and in this case you can invoke the rendering modules directly. Additionally you can now invoke rendering module branching to output graphics into different rendering modules simultaneously. A classic use-case, where an application invokes vectorization modules to draw existing databases, doesn’t change and can be used as with previous ODA SDK versions, but the new module structure gives additional possibilities for new applications.
This article describes the set of visualization modules and libraries, how to correctly link applications with them, and how to load these modules.
Visualization Modules Map
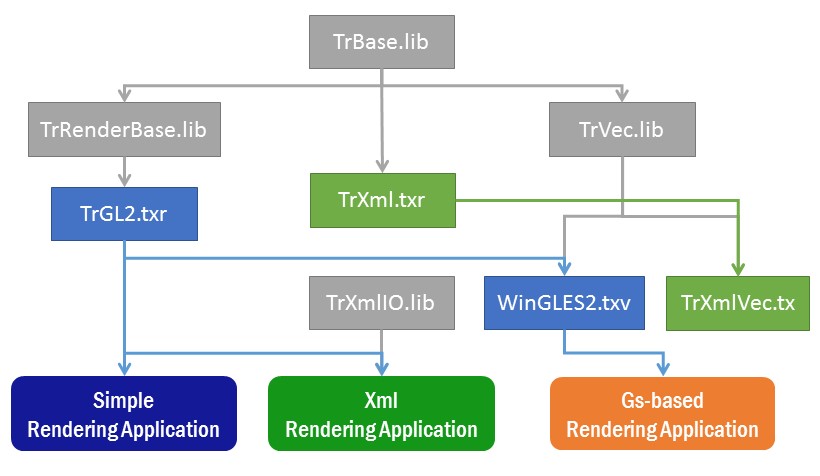
- Gray rectangles — Required static libraries.
- Blue rectangles — Main modules required for visualization applications.
- Green rectangles — Optional modules required for exporting rendering streams to XML format.
- Rounded rectangles — Different final applications.
TrRenderBase.lib is required for building rendering modules only. Export modules (like XML export in this diagram) don’t require this library for their functionality.
Changes to ODA SDKs Beginning with Version 20.2
- TrGL2.lib static library becomes TrGL2.txr rendering module.
- TrRenderBase.lib is a new static library, invoked by OpenGL ES 2.0 renderer and vectorizer.
- TrXml.txr is a new rendering module, required for exporting rendering streams into XML file format.
- XmlGLES2.txv vectorization module becomes TrXmlVec.tx extension module.
If your final application invokes only OpenGL ES 2.0 rendering, take into account only the first two items. If your final application invokes XML export functionality, take into account the last two items.
Note: Don’t forget that in static library configurations, all dynamic libraries (.tx, .txv, .txr) become static libraries that should be linked with the final application. Because the WinGLES2 vectorization module now internally invokes the TrGL2 rendering module, in a static library configuration the application additionally should register the new rendering module in the static modules map (this change is described in this article later in “Static Libraries Configuration”).
Visualization Modules Description
Module name | Module type | Description |
---|---|---|
TrBase.lib | Static Library | Library that contains general functions and classes that are used in all visualization-related libraries and modules. |
TrRenderBase.lib | Static Library | Library that contains general functions and classes for building rendering modules. |
TrVec.lib | Static Library | Basic library that contains all required functionality for building vectorization modules. |
TrGL2.txr | Rendering Module | OpenGL ES 2.0 renderer. |
WinGLES2.txv | Vectorization Module | OpenGL ES 2.0 vectorization module (invoke TrGL2.txr for rendering in Gs-based applications). |
TrXml.txr | Rendering Module | XML export module. |
TrXmlVec.tx | Extension | Vectorization module that invokes TrXml.txr rendering module for exporting vectorized data to XML format. |
TrXmlIO.lib | Static Library | Library for applications that open and process vectorized data from XML format, for example, load XML data and render it using another rendering module. |
Dynamic Library Configurations
Rendering Modules
New rendering modules are defined in the OdModuleNames.h header file, which is available for all ODA SDK based applications:
#define OdTrGL2ModuleName L"TrGL2.txr"
#define OdTrXmlModuleName L"TrXml.txr"
Rendering modules can be loaded using the following call (which uses a dynamic modules linker):
OdTrRndRenderModulePtr pRenderModule = ::odrxDynamicLinker()->loadModule(OdTrGL2ModuleName);
Vectorization Modules
The same definitions are available for vectorization modules:
#define OdWinGLES2ModuleName L"WinGLES2.txv"
#define OdTrXmlVecModuleName L"TrXmlVec.tx"
These modules can be loaded just like any other vectorization modules (there are no changes from previous ODA SDK versions):
OdGsModulePtr pGs = ::odrxDynamicLinker()->loadModule(OdWinGLES2ModuleName);
Static Library Configurations
Linking Libraries
To link with TrGL2 renderer, add the following set of libraries to the related project’s CMakeLists.txt file:
${TR_TXR_GL2_LIB} ${TR_RENDER_LIB} ${TR_BASE_LIB}
Add the following set of libraries to the linker for linking with the WinGLES2 vectorization module:
${TD_TXV_GLES2_LIB} ${TR_TXR_GL2_LIB} ${TR_RENDER_LIB} ${TR_VEC_LIB} ${TR_BASE_LIB}
A special CMake definition is available to avoid adding this long list of libraries. To link all required libraries for WinGLES2 vectorization, you can use the $(TD_TXV_GLES2_LIBS} CMake definition only:
set(TD_TXV_GLES2_LIBS ${TD_TXV_GLES2_LIB} ${TR_TXR_GL2_LIB} ${TR_RENDER_LIB} ${TR_VEC_LIB} ${TR_BASE_LIB})
There is one more definition that was kept for older projects; the definition links with the WinGLES2 vectorization module that invokes the obsolete TrGL2.lib static library:
# for compatibility with old CMakeLists
set(TR_GL2_LIB ${TR_TXR_GL2_LIB} ${TR_RENDER_LIB})
So old projects, if they don’t invoke non-standard features in CMake, don’t currently require special changes in the related CMakeLists.txt file. But it is better to avoid using the obsolete $(TR_GL2_LIB} definition, since it might be removed in the future.
For libraries that require linking with the TrXml.txr rendering module (XML export):
${TR_TXR_XML_LIB} ${TR_BASE_LIB}
For linking with TrXmlVec.tx, your application requires the following libraries in the linked libraries list:
${TR_XML_VEC_LIB} ${TR_TXR_XML_LIB} ${TR_VEC_LIB} ${TR_BASE_LIB}
Static Modules Map
New rendering modules should be registered in the static modules map too, if the application invokes the WinGLES2 vectorization module:
ODRX_DECLARE_STATIC_MODULE_ENTRY_POINT(OdTrGL2RenderModule);
ODRX_DECLARE_STATIC_MODULE_ENTRY_POINT(GLES2Module);
ODRX_BEGIN_STATIC_MODULE_MAP()
ODRX_DEFINE_STATIC_APPLICATION(OdTrGL2ModuleName, OdTrGL2RenderModule)
ODRX_DEFINE_STATIC_APPMODULE(OdWinGLES2ModuleName, GLES2Module)
ODRX_END_STATIC_MODULE_MAP()
The WinGLES2.txv vectorization module entry point is GLES2Module, as with previous versions. For TrGL2.txr, the rendering module entry point is OdTrGL2RenderModule.
It is the same with XML export applications:
ODRX_DECLARE_STATIC_MODULE_ENTRY_POINT(OdTrXmlRenderModule);
ODRX_DECLARE_STATIC_MODULE_ENTRY_POINT(TrXmlModule);
ODRX_BEGIN_STATIC_MODULE_MAP()
ODRX_DEFINE_STATIC_APPLICATION(OdTrXmlModuleName, OdTrXmlRenderModule)
ODRX_DEFINE_STATIC_APPLICATION(OdTrXmlVecModuleName, TrXmlModule)
ODRX_END_STATIC_MODULE_MAP()
The TrXmlVec.tx (previously XmlGLES2.txv) vectorization module entry point is TrXmlModule, as with previous versions. For TrXml.txr, the rendering module entry point is OdTrXmlRenderModule.